JQuery getJSON - ajax parseerror
Solution 1
The JSON string you have is an array with 1 object inside of it, so to access the object you have to access the array first. With a json.php that looks like this:
[
{
"iId": "1",
"heading": "Management Services",
"body": "<h1>Program Overview</h1><h1>January 29, 2009</h1>"
}
]
I just tried this
$.getJSON("json.php", function(json) {
alert(json[0].body); // <h1>Program Overview</h1><h1>January 29, 2009</h1>
alert(json[0].heading); // "Management Services"
alert(json[0].iId); // "1"
});
I also tried this:
$.get("json.php", function(data){
json = eval(data);
alert(json[0].body); // <h1>Program Overview</h1><h1>January 29, 2009</h1>
alert(json[0].heading); // "Management Services"
alert(json[0].iId); // "1"
});
And they both worked fine for me.
Solution 2
If anyone is still having problems with this it's because your response needs to be a JSON string and content-type "application/json".
Example for HTTP in asp.net (c#):
public void ProcessRequest(HttpContext context)
{
context.Response.ContentType = "application/json";
context.Response.Write("{ status: 'success' }");
}
hth,
Matti
Solution 3
Disabling Firebug Lite fixed this problem for me.
Bug with combination of: jQuery 1.4, ajax/json, Firebug Lite and IE 8
Solution 4
Did you try XML-encoding the HTML (i.e. <H1>)?
Solution 5
You could have it return as text and then parse it with the json.org parser
To see if it works any differently
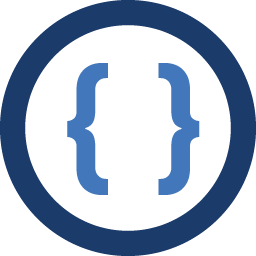
Admin
Updated on December 01, 2020Comments
-
Admin over 3 years
I've tried to parse the following json response with both the JQuery getJSON and ajax:
[{"iId":"1","heading":"Management Services","body":"<h1>Program Overview</h1><h1>January 29, 2009</h1>"}]
I've also tried it escaping the "/" characters like this:
[{"iId":"1","heading":"Management Services","body":"<h1>Program Overview <\/h1><h1>January 29, 2009<\/h1>"}]
When I use the getJSON it dose not execute the callback. So, I tried it with JQuery ajax as follows:
$.ajax({ url: jURL, contentType: "application/json; charset=utf-8", dataType: "json", beforeSend: function(x) { if(x && x.overrideMimeType) { x.overrideMimeType("application/j-son;charset=UTF-8"); } }, success: function(data){ wId = data.iId; $("#txtHeading").val(data.heading); $("#txtBody").val(data.body); $("#add").slideUp("slow"); $("#edit").slideDown("slow"); },//success error: function (XMLHttpRequest, textStatus, errorThrown) { alert("XMLHttpRequest="+XMLHttpRequest.responseText+"\ntextStatus="+textStatus+"\nerrorThrown="+errorThrown); } });
The ajax hits the error ans alerts the following:
XMLHttpRequest=[{"iId":"1","heading":"Management Services","body":"<h1>Program Overview </h1><h1>January 29, 2009</h1>"}] textStatus=parseerror errorThrown=undefined
Then I tried a simple JQuery get call to return the JSON using the following code:
$.get(jURL,function(data){ var json = eval("("+data+");"); wId = json.iId; $("#txtHeading").val(json.heading); $("#txtBody").val(json.body); $("#add").slideUp("slow"); $("#edit").slideDown("slow"); })
The .get returns the JSON, but the eval comes up with errors no matter how I've modified the JSON (content-type header, other variations of the format, etc.)
What I've come up with is that there seem to be an issue returning the HTML in the JSON and getting it parsed. However, I have hope that I may have missed something that would allow me to get this data via JSON. Does anyone have any ideas?