Jquery jsonp response error - Callback was not called
Solution 1
There are a few issues with your $.ajax
call.
$.ajax({
url: url + '?callback=?',
// this is not needed for JSONP. What this does, is force a local
// AJAX call to accessed as if it were cross domain
crossDomain: true,
// JSONP can only be GET
type: "POST",
data: {key: key},
// contentType is for the request body, it is incorrect here
contentType: "application/json; charset=utf-8;",
// This does not work with JSONP, nor should you be using it anyway.
// It will lock up the browser
async: false,
dataType: 'jsonp',
// This changes the parameter that jQuery will add to the URL
jsonp: 'callback',
// This overrides the callback value that jQuery will add to the URL
// useful to help with caching
// or if the URL has a hard-coded callback (you need to set jsonp: false)
jsonpCallback: 'jsonpCallback',
error: function(xhr, status, error) {
console.log(status + '; ' + error);
}
});
You should be calling your url like this:
$.ajax({
url: url,
data: {key: key},
dataType: 'jsonp',
success: function(response) {
console.log('callback success');
},
error: function(xhr, status, error) {
console.log(status + '; ' + error);
}
});
JSONP is not JSON. JSONP is actually just adding a script tag to your <head>
. The response needs to be a JavaScript file containing a function call with the JSON data as a parameter.
JSONP is something the server needs to support. If the server doesn't respond correctly, you can't use JSONP.
Please read the docs: http://api.jquery.com/jquery.ajax/
Solution 2
var url = "https://status.github.com/api/status.json?callback=apiStatus";
$.ajax({
url: url,
dataType: 'jsonp',
jsonpCallback: 'apiStatus',
success: function (response) {
console.log('callback success: ', response);
},
error: function (xhr, status, error) {
console.log(status + '; ' + error);
}
});
Try this code.
Also try calling this url directly in ur browser and see what it exactly returns, by this way You can understand better what actually happens :).
Solution 3
The jsonpCallback
parameter is used for specifying the name of the function in the JSONP response, not the name of the function in your code. You can likely remove this; jQuery will handle this automatically on your behalf.
Instead, you're looking for the success
parameter (to retrieve the response data). For example:
$.ajax({
url: url,
crossDomain: true,
type: "POST",
data: {key: key},
contentType: "application/json; charset=utf-8;",
async: false,
dataType: 'jsonp',
success: function(data){
console.log('callback success');
console.log(data);
}
error: function(xhr, status, error) {
console.log(status + '; ' + error);
}
});
You can also likely remove the other JSONP-releated parameters, which were set to jQuery defaults. See jQuery.ajax
for more information.
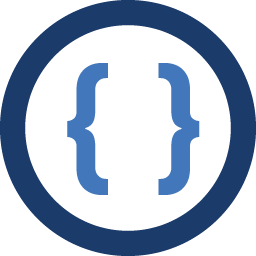
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'm trying to get some information from a different domain, the domain allows only jsonp call - others get rejected. How can I get the content instead of execution? Because I get an error in response. I don't need to execute it, I just need it in my script. In any format (the response is json but js doesn't understand it). I can't affect on that domain so it's impossible to change something on that side. Here's my code:
$.ajax({ url: url + '?callback=?', crossDomain: true, type: "POST", data: {key: key}, contentType: "application/json; charset=utf-8;", async: false, dataType: 'jsonp', jsonp: 'callback', jsonpCallback: 'jsonpCallback', error: function(xhr, status, error) { console.log(status + '; ' + error); } }); window.jsonpCallback = function(response) { console.log('callback success'); };