jQuery pause function on hover?
Solution 1
Something like this would work assuming interval
is defined in an outer scope:
$('.slideshow img').hover(function() {
interval = clearInterval(interval);
}, function() {
interval = setInterval(flip, 5000);
});
Solution 2
(function () {
var imgs = $('#your_div img'), index = 0, interval,
interval_function = function () {
imgs.eq(index).hide();
index = (index + 1) % imgs.length;
imgs.eq(index).show();
};
imgs.eq(0).show();
interval = setInterval(interval_function, 5000);
$('#your_div').hover(function () {
clearInterval(interval);
}, function () {
interval = setInterval(interval_function, 5000);
});
}());
Example: http://jsfiddle.net/Zq7KB/3/
I reused some old code I wrote for a question the other day, but I figured it didn't matter that much. The trick is to store your interval in a variable that you keep in the background. Then, when you hover over the container, clear the interval. When you hover out of the container, re-set the interval. To get a better feel of how this works, change those 5000
s to 1000
s so it passes more quickly for testing.
Hope this helps.
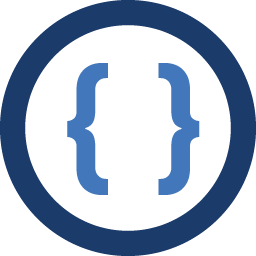
Admin
Updated on June 11, 2022Comments
-
Admin almost 2 years
I have a jQuery/JS function that is using
setInterval
to loop through some image slides I have. It just flips through every 5 seconds...Now I want it to pause if my mouse is hovered over it. How do I go about doing that on the setInterval function?
var current = 1; function autoAdvance() { if (current == -1) return false; jQuery('#slide_menu ul li a').eq(current % jQuery('#slide_menu ul li a').length).trigger('click', [true]); current++; } // The number of seconds that the slider will auto-advance in: var changeEvery = jQuery(".interval").val(); if (changeEvery <= 0) { changeEvery = 10; } var itvl = setInterval(function () { autoAdvance() }, changeEvery * 1000);
-
Jacob Relkin over 13 yearsI was almost going to point out that
interval_function
was global, but then I saw the comma from the previous line... -
Admin over 13 yearsOk i tried it but i didn't expect it to work because my code is not exactly orthodox...please see my updated post on the code i am using to flip the images...