jQuery - select div at same level
25,433
Solution 1
This should do it.
.closest
will go up through the parents until it finds a match. Then from that you can .find
the target div that you are looking for.
$(".viewPrices").click(function () {
$(this).closest('.listcontainer').find('.list').toggleClass('visible');
});
here is an updated fiddle: http://jsfiddle.net/n264v/2/
Solution 2
Using
$(this).parent().parent().children("div:eq(0)").toggleClass('visible');
This will select the parent div of the parent div where the button is.
Solution 3
Edited your JSFiddle: http://jsfiddle.net/n264v/3/
Also, the following code works too:
$(".viewPrices").click(function () {
$(".viewPrices").parent().siblings('.list').toggleClass('visible');
});
For this HTML:
<div class="container">
<div class="box">
<h2>Langtidsparkering</h2>
<div class="content">
Lorem ipsum dolor sit amet..
</div>
</div>
<div class="listcontainer">
<div class="list"> (THIS DIV SHOULD GET A CLASS "VISIBLE", WHEN THE BUTTON IS CLICKED)
</div>
<div class="listbar">
<button class="viewPrices" type="submit" title="Open">Se priser<span></span </button>
</div>
</div>
</div>
Took the liberty to add the following css:
.list{
display:none;
}
.visible{
display:block !important;
}
Solution 4
First of there is no div with the class "listaccordion" so you will never find a match.
You can use:
$(".viewPrices").click(function () {
$(this).parent(".listbar").siblings(".list").toggleClass("visible");
}
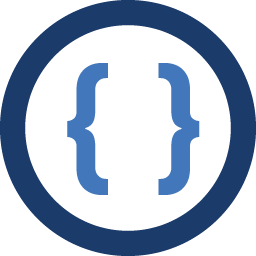
Author by
Admin
Updated on March 01, 2020Comments
-
Admin about 4 years
I want to select a specific div, when clicking on a button... The only issue is, it has to be the div of the buttonClicked's parent div... Sample:
<div class="container"> <div class="box"> <h2>Langtidsparkering</h2> <div class="content"> Lorem ipsum dolor sit amet.. </div> </div> <div class="listcontainer"> <div class="list"> (THIS DIV SHOULD GET A CLASS "VISIBLE", WHEN THE BUTTON IS CLICKED) </div> <div class="listbar"> <button class="viewPrices" type="submit" title="Open">Se priser<span></span </button> </div> </div> </div>
Code:
$(".viewPrices").click(function () { $(".viewPrices").parents('.listaccordion .list').toggleClass('visible'); });
Any suggestions ? :-)
-
Admin about 10 yearsActually likes this one, but both divs with class .list opened when clicking the button... Hm.. weird..
-
mathius1 almost 4 yearsThis code will toggle EVERY element with the class "list"