Jquery UI autocomplete - images IN the results overlay, not outside like the demo
The recommended way to change the display of list items in the suggestion list for autocomplete is to override the _renderItem
method. Inside this method you must do several things:
- Append an
li
to the passed inul
. Theli
must include ana
tag. - Associate the proper data with that
li
- Return that
li
.
Apart from this, you can perform any custom formatting logic you'd like. Here's how I would update your code:
$("input#autocomplete").autocomplete({
delay: 10,
minLength: 0,
source: updates,
select: function( event, ui ) {
window.location.href = ui.item.value;
}
}).data("autocomplete")._renderItem = function (ul, item) {
return $("<li />")
.data("item.autocomplete", item)
.append("<a><img src='" + item.icon + "' />" + item.label + "</a>")
.appendTo(ul);
};
As you can see, adding an img
tag was as easy as placing it inside of the a
tag mentioned above. Keep in mind you can also apply CSS rules to items that you add as well.
Here's a complete example that uses StackOverflow's API for searching users, and shows their avatars to the left of them.
Update: As of jQueryUI 1.10, you need to access widget data for autocomplete using .data("uiAutocomplete")
(thanks for noticing the problem @Danny). With that in mind, here's an example working in 1.10:
$("input#autocomplete").autocomplete({
delay: 10,
minLength: 0,
source: updates,
select: function( event, ui ) {
window.location.href = ui.item.value;
}
}).data("uiAutocomplete")._renderItem = function (ul, item) {
return $("<li />")
.data("item.autocomplete", item)
.append("<a><img src='" + item.icon + "' />" + item.label + "</a>")
.appendTo(ul);
};
Example: http://jsfiddle.net/K5q5U/249/
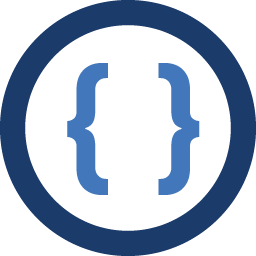
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I have jQueryUI code for autocompleting that follows....
$(function() { var updates = [ { value: "URL", label: "some text", icon: "jqueryui_32x32.png"}; ]; $("input#autocomplete").autocomplete({ delay: 10, minLength: 0, source: updates, select: function( event, ui ) { window.location.href = ui.item.value; } }); });
And it essentially produces a site search where the results are direct links. I would like to add images that are stored locally to the results to greater imitate facebook. I've had no luck and seen questions before directing users to the "custom data" demo. Here is one such question...
How do I add images to results of this JQueryUI autocomplete plugin?
I'm aware or this demo...
http://jqueryui.com/demos/autocomplete/#custom-data
..but there is NO guidance as to how one would go about do this IN DETAIL about formatting the results overlay itself / putting images IN the overlay. I'm realy at a loss here. I've dissected this demo all day with nothing to show for it. This should be easy because all the data and images are local. They're all in the same folder as this search. There's no PHP or database stuff to deal with here.....
I really, truly hate jQuery UI because of how poorly documented it is, and how unnecessarily complicated it is.... All the customization stuff is in multiple files, etc.... I used to use JÖRN ZAEFFERER's plugin which accepted simple HTML tags. It worked just fine until IE8......
Any insights into this are greatly, greatly appreciated.
-
Danny about 11 yearsThis does not seem with jquery ui 1.10.2.
.data("autocomplete")
is undefined. I updated your fiddle. Any ideas on how to fix it? -
Andrew Whitaker about 11 years@Danny: Sorry about that, try
.data("uiAutocomplete")
instead. Here's the working example: jsfiddle.net/K5q5U/249 -
Mubasshir Pawle almost 11 years@AndrewWhitaker it was great solution and it worked for me until I used it after ajax call. though autocomplete dropdown is coming but not images. I feel there is an issue with data
-
Andrew Whitaker almost 11 years@MubasshirNazirPawle: Might be best to ask a new question with more information.
-
andreybavt over 8 yearsStackOverflow API v1 is no longer available either, the url that should be used in the example is api.stackexchange.com/2.2/…