jQuery UI Datepicker set display month
10,580
Solution 1
Assuming you have mm/dd/yyyy mask, like in the demo you could do like this:
UPD2. Using Datepicker API
function ChangeMonth(month)
{
var initialVal = $('#datepicker').datepicker('getDate')
curMonth = date.getMonth(),
if(month > 0 && curMonth < 12){
initialVal.setMonth(curMonth + 1)
}
if(month < 0 && curMonth > 1) {
initialVal.setMonth(curMonth - 1)
}
$('#datepicker').datepicker('setDate', initialVal);
}
The calling ChangeMonth(1)
or ChangeMonth(-1)
should change the date in your datapicker field.
UPD1. If you're using inlined version
You can just "click" buttons with jQuery click event:
$('#datepicker .ui-datepicker-prev').click() // month back
$('#datepicker .ui-datepicker-next').click() //month forward
Solution 2
The facts:
-
$("#datepicker").datepicker("setDate", date)
should be used for set "selected date". As a side effect, it will also change the "display month". but use it only for setting "display month" is not a good practice. -
$('#datepicker .ui-datepicker-prev').click()
and$('#datepicker .ui-datepicker-next').click()
should be used for setting "display month" and it does not have side effects. But be aware, it is slow if you set the "display months" a few years away. - There is no function to get current "display month" directly.
Based on the facts, here is the solution:
var currentDate = new Date ();
var currentMonth = new Date (currentDate.getFullYear(), currentDate.getMonth(), 1);
//Keep currentMonth sync with "display month"
$("#datepicker").datepicker({
onChangeMonthYear: function (year, month, inst) {
currentMonth = new Date(year, month - 1, 1);
//JavaScript used 0...11 for months. Jquery UI used 1...12 for months.
},
});
function ChangeMonth(month)
{
while (currentMonth < month) {
$('#datepicker .ui-datepicker-next').click();
};
while (currentMonth > month) {
$('#datepicker .ui-datepicker-prev').click();
};
};
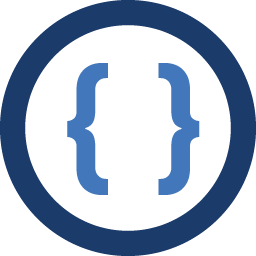
Author by
Admin
Updated on August 31, 2022Comments
-
Admin over 1 year
I need to set the display month on a datepicker in the click event of another control. Is that possible? I can't use the setDate method since that will set a specific date, whereas I'm trying to emulate the prev/next month buttons on the datepicker.
EDIT: I'm using the inline datepicker https://jqueryui.com/datepicker/#inline
html:
<input type="button" onclick="ChangeMonth(1);" />
javascript:
function ChangeMonth(month) { //set month on datepicker $("#DatePicker").??? }
-
Admin almost 9 yearssorry, I should have specified I'm using the inline example, jqueryui.com/datepicker/#inline
-
shershen almost 9 yearshmmm, yes that would have been handy to know )) Check the updated the post!
-
dmeglio almost 9 yearsYou should never write code like this. It's a bad idea if you ever wind up localizing your code to work in other locales. All of a sudden, this breaks. Rely on the APIs your system provides.
-
shershen almost 9 years@dman2306 you're right in general. Also jQueryUI Datepicker widget has 'dateFormat' option and if it's set then date will be displayed only in that way, not considering the locale. Rewrote that part.
-
Admin almost 9 yearswow, i hadn't thought of your inline solution! in my case I need to set it using the month parameter like so, month + "dayandyear". If i could get the current displayed month then I would be able to compare it to that and use the prev/next buttons. Do you know if that is possible without having a date selected?
-
Admin almost 9 yearsI don't think this will work with an inline datepicker. Sorry I didn't specify that earlier.
-
Brino almost 9 yearsi updated the fiddle. It works fine with the inline date picker. jsfiddle.net/seadonk/uh9g9sn4
-
Admin almost 9 yearsthis works great, except it's setting a specific date when the month is changed, which I'm trying to avoid.
-
Brino almost 9 yearswhen the month is changed it simply decrements or increments the month number, the day stays the same, it needs setdate to do this. This seems to fit your description of prev. month and next month. What do you need it to do specifically? and why is this not sufficient?
-
Anastasios Moraitis over 2 yearsSaved the day! Great solution!