jQuery Validation plugin: How to force a certain phone number format ###-###-####
Solution 1
@Sparky's suggestion is good if you are a little flexible, but just in case you want just that format, you can add a custom rule:
$.validator.addMethod('customphone', function (value, element) {
return this.optional(element) || /^\d{3}-\d{3}-\d{4}$/.test(value);
}, "Please enter a valid phone number");
$(document).ready(function () {
$("#myform").validate({
rules: {
field1: 'customphone'
}
});
});
Example: http://jsfiddle.net/kqczf/16/
You can easily make this into a custom class rule. This way you could just add a class to each input that you want to have the rule and possibly omit the rules object from the validate
call:
$(document).ready(function () {
$("#myform").validate();
});
<input type="text" name="field1" id="field1" class="required customphone" />
Example: http://jsfiddle.net/kqczf/17/
Solution 2
Simply use the phoneUS
rule included in the jQuery Validate plugin's additional-methods.js
file.
DEMO: http://jsfiddle.net/c9zy9/
$(document).ready(function () {
$('#myform').validate({ // initialize the plugin
rules: {
phone_number: {
required: true,
phoneUS: true
}
}
});
});
Alternatively, instead of including the entire additional-methods.js
file, you can just pull out the phoneUS
method.
DEMO: http://jsfiddle.net/dSz5j/
$(document).ready(function () {
jQuery.validator.addMethod("phoneUS", function (phone_number, element) {
phone_number = phone_number.replace(/\s+/g, "");
return this.optional(element) || phone_number.length > 9 && phone_number.match(/^(\+?1-?)?(\([2-9]\d{2}\)|[2-9]\d{2})-?[2-9]\d{2}-?\d{4}$/);
}, "Please specify a valid phone number");
$('#myform').validate({ // initialize the plugin
rules: {
phone_number: {
required: true,
phoneUS: true
}
}
});
});
Solution 3
Check out the jQuery Masked Input Plugin. It allows you to mask the input with something like this:
$("#phone_number").mask("999-999-9999");
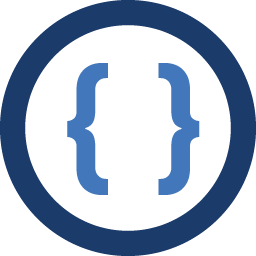
Admin
Updated on June 16, 2022Comments
-
Admin almost 2 years
I have jQuery Validation plugin on a page. When someone types the phone number into the form field, I want the validator to only recognize a certain format:
###-###-####
I see this in the JavaScript:
phone_number: { required: true, number: true },
Can I add something there to specify the required format for the phone number? I found something about adding the jQuery Mask plugin, but if I can avoid it, I'd rather not add to the page weight. (Plus...surely there must exist some way to validate only a certain format.)
-
Andrew Whitaker about 11 yearsThis isn't exactly what the user wants--the dashes are optional and +1 is also allowed.
-
Sparky about 11 years@AndrewWhitaker, I appreciate that but sometimes the user doesn't even know these rules already exist.
-
Admin about 11 yearsIn this case, I wasn't aware that the rule existed, so that you for that. But we do need it to be that very exact format; and for our current purposes, they're telling me that they don't care about non-US phone numbers. (Which I think is going to bite them in the backside, but...there ya go. They've heard my objections, they've given their decision.)
-
Admin about 11 yearsThank you; but I'd like to try and accomplish this without adding any additional plugins.
-
Admin about 11 yearsThanks @Andrew - that looks like what I'm trying to do. Do I add that into the document header, and just make sure that it calls out the proper form ID? (And for cases where this is a global include, and I want to add this action to a few different form IDs, can I have multiple form IDs? And for the field1 - does that need to match the field's class name, or id, or both?)
-
Andrew Whitaker about 11 yearsYou could use a class rule, I'll update my answer. If you do it for each form, the rule key (
field1
) needs to match up with the field name. Sounds like using a class rule is what you want though, I'll update the answer. -
Andrew Whitaker about 11 years@Leigh: You can put the
$.validator.addMethod
and $.validator.addClassRules` lines in your document header. Your call to.validate
should occur when the document is ready. -
Sparky about 11 yearsYou do not need the
addClassRules
method to simply use this custom rule withinclass
. See: jsfiddle.net/kqczf/18 -
Andrew Whitaker about 11 years@Sparky: Thanks for the tip, I didn't realize it would work without it.
-
Sparky about 11 yearsYou'd need the
addClassRules
method if you wanted to assign a "compound" rule to a class name: jsfiddle.net/kqczf/19 -
Fabio Pigagnelli about 10 yearsVery useful, thank you. It was exactly what I was looking for.