JSON Post with Customized HTTPHeader Field
Solution 1
What you posted has a syntax error, but it makes no difference as you cannot pass HTTP headers via $.post()
.
Provided you're on jQuery version >= 1.5, switch to $.ajax()
and pass the headers
(docs) option. (If you're on an older version of jQuery, I will show you how to do it via the beforeSend
option.)
$.ajax({
url: 'https://url.com',
type: 'post',
data: {
access_token: 'XXXXXXXXXXXXXXXXXXX'
},
headers: {
Header_Name_One: 'Header Value One', //If your header name has spaces or any other char not appropriate
"Header Name Two": 'Header Value Two' //for object property name, use quoted notation shown in second
},
dataType: 'json',
success: function (data) {
console.info(data);
}
});
Solution 2
if one wants to use .post() then this will set headers for all future request made with jquery
$.ajaxSetup({
headers: {
'Content-Type': 'application/json',
'Accept': 'application/json'
}
});
then make your .post() calls as normal.
Solution 3
I tried as you mentioned, but only first parameter is going through and rest all are appearing in the server as undefined
. I am passing JSONWebToken
as part of header.
.ajax({
url: 'api/outletadd',
type: 'post',
data: { outletname:outletname , addressA:addressA , addressB:addressB, city:city , postcode:postcode , state:state , country:country , menuid:menuid },
headers: {
authorization: storedJWT
},
dataType: 'json',
success: function (data){
alert("Outlet Created");
},
error: function (data){
alert("Outlet Creation Failed, please try again.");
}
});
Solution 4
Just wanted to update this thread for future developers.
JQuery >1.12 Now supports being able to change every little piece of the request through JQuery.post ($.post({...}). see second function signature in https://api.jquery.com/jquery.post/
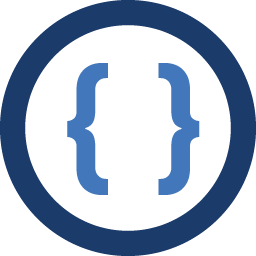
Admin
Updated on July 08, 2022Comments
-
Admin almost 2 years
I have inherited some code that will eventually be part of an API call. Based on the existing code, the call is a post to retrieve JSON code with an access_token. While this would normally be simple and like every other API out there, this code requires that there be a customized httpheader field for the client secret.
I was able to make this work in Objective C with URLRequest, etc. but now that I am creating the call for a web component, I have been roadblocked.
I am using a pretty standard jquery post
$.post('https://url.com', {access_token:'XXXXXXXXXXXXXXXXXXX', function(data){ console.info(data); }, 'json');
With a HTTP-EQUIV in the header. But the post never retrieves data and the server itself doesn't recognized that any call was made (even an incomplete one).
I may have to scrap this code and start over, but if anyone has encountered this problem before, please offer any insight.
-
Admin over 12 yearsThank you so much! You saved me hours of frustration. Now, the object is being pulled in Safari, but not in Firefox or Chrome. Is this a known, related problem? If so, I would love your insight. If not, I'll figure it out.
-
JAAulde over 12 yearsWhen using Chrome and Firefox, what do you see (via Developer Tools or Firebug) being sent to the server, and what do you see returned? What debugging do you have at the server to see if the request is incoming and proper?
-
Admin over 12 yearsNo errors appear in Firebug at all, but there is no return. In the Firefox Web Console I see this: 'OPTIONS https://[url.com] [HTTP/1.1 200 OK 441ms]' and in Chrome Developer tools I see this: XMLHttpRequest cannot load 'url.com. Origin null is not allowed by Access-Control-Allow-Origin.' The server shows that a request came in. If the request were improper it would log an error message.
-
JAAulde over 12 yearsWell, without access to the code or system, I won't be able to help too much. The AJAX request is being made to the same domain as the one which served the page, correct?
-
Admin over 12 yearsYep, but I think I figured out a solution. Thanks for all your help!
-
vgoklani almost 8 years"it makes no difference as you cannot pass HTTP headers via $.post()"