Keep Secret Keys Out
Solution 1
Store your local_settings.py
data in a file encrypted with GPG - preferably as strictly key=value
lines which you parse and assign to a dict (the other attractive approach would be to have it as executable python, but executable code in config files makes me shiver).
There's a python gpg module so that's not a problem. Get your keys from your keyring, and use the GPG keyring management tools so you don't have to keep typing in your keychain password. Make sure you are reading the data straight from the encrypted file, and not just creating a decrypted temporary file which you read in. That's a recipe for fail.
That's just an outline, you'll have to build it yourself.
This way the secret data remains solely in the process memory space, and not in a file or in environment variables.
Solution 2
Ideally, local_settings.py
should not be checked in for production/deployed server. You can keep backup copy somewhere else, but not in source control.
local_settings.py
can be checked in with development configuration just for convenience, so that each developer need to change it.
Does that solve your problem?
Solution 3
I doing my Django projects using Windows 7 and Powershell, so for me it was slightly different to set the environment variable. Once it was set though, I just did the following in my settings.py
file:
import os
SECRET_KEY = os.environ["SOME_SECRET_KEY"]
To set a environment variable in Windows using PowerShell follow the instructions in the link below:
http://technet.microsoft.com/en-us/library/ff730964.aspx
Solution 4
The original question was about how to keep secrets in environment variables. This is discussed extensively in the book Two Scoops of Django. Below is a summary of what they said, followed by a caveat about using this technique.
Starting on page 48 (Section 5.3) of the edition for 1.11:
Every operating system supported by Django (and Python) provides the easy capability to create environment variables.
Here are the benefits of using environment variables for secret keys:
- Keeping secrets out of settings allows you to store every settings file in version control without hesitation. All of your Python code really should be stored in version control, including your settings.
- Instead of each developer maintaining their own copy-and-pasted version of local_settings.py.example for development, everyone shares the same version-controlled settings/local.py .
- System administrators can rapidly deploy the project without having to modify files containing Python code.
- Most platforms-as-a-service recommend the use of environment variables for configuration and have built-in features for setting and managing them.
On the following page, the book continues:
Before you begin setting environment variables, you should have the following:
- A way to manage the secret information you are going to store.
- A good understanding of how bash settings work on servers, or a willingness to have your project hosted by a platform-as-a-service.
They describe how to set the environment variables locally and in production (with Heroku as an example--you will need to check if you are using a different host this is just one possibility):
How To Set Environment Variables Locally
export SOME_SECRET_KEY=1c3-cr3am-15-yummyHow To Set Environment Variables in Production
heroku config:set SOME_SECRET_KEY=1c3-cr3am-15-yummy
Finally, on page 52 they give instructions for how to access the key. For instance you could put the first two lines below in your settings file to replace the raw key string that is put there by default:
>>> import os >>> os.environ['SOME_SECRET_KEY'] '1c3-cr3am-15-yummy'
This snippet simply gets the value of the SOME_SECRET_KEY environment variable from the operating system and saves it to a Python variable called SOME_SECRET_KEY.
Following this pattern means all code can remain in version control, and all secrets remain safe.
Note this will not work in some cases, for instance if you are using an Apache server. To deal with situations where this pattern will not work, you should see Section 5.4 of their book ('When You Can't Use Environment Variables'). In that case, they recommend use a secret file.
As of late 2017, this technique of storing secrets in your environment variables is the recommended best practice in Two Scoops and in the Twelve Factor App design pattern. It is also recommended at the Django docs. However, there are some security risks: if some developer, or some code, has access to your system, they will have access to your environment variables and may inadvertently (or advertently) make them public. This point was made by Michael Reinsch here:
http://movingfast.io/articles/environment-variables-considered-harmful/
Solution 5
I wrote a getcreds() function which gets the secret key from a file. I keep the file in a place accessible to www-data so wherever I need credentials in settings.py I just make the call to getcreds() passing in the filename as an argument. It returns a list of all lines in the file and bingo I have the hidden secrets. Here is the code ...
from __future__ import unicode_literals, absolute_import
import os
def getcreds(fname, project, credsroot='/var/www/creds', credsdir=None):
""" return a list of userid and password and perhaps other data """
if credsdir is None:
credsdir = os.path.join(credsroot, project)
creds = list()
fname = os.path.join(credsdir, fname).replace("\\", "/")
with open(fname, 'r') as f:
for line in f:
# remove leading/trailing whitespace and append to list
creds.append(line.strip())
assert creds, "The list of credentials is empty"
return creds
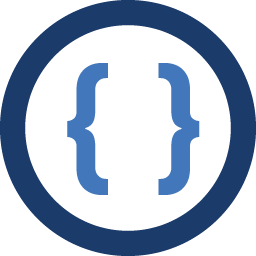
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
One of the causes of the local_settings.py anti-pattern is that putting SECRET_KEY, AWS keys, etc.. values into settings files has problem:
- Secrets often should be just that: secret! Keeping them in version control means that everyone with repository access has access to them.
My question is how to keep all keys as secret?