Key (enum) to string
11,860
Solution 1
Use a Dictionary<TKey, TValue>
:
Class-level:
private readonly Dictionary<string, string> operations = new Dictionary<string, string>;
public ClassName() {
// put in constructor...
operations.Add("Subtract", "-");
// etc.
}
In your method, just use operations[e.Key.ToString()]
instead.
Edit: Actually, for more efficiency:
private readonly Dictionary<System.Windows.Input.Key, char> operations = new Dictionary<System.Windows.Input.Key, char>;
public ClassName() {
// put in constructor...
operations.Add(System.Windows.Input.Key.Subtract, '-');
// etc.
}
In your method, just use operations[e.Key]
instead.
Solution 2
Well, you can use a cast if you want to convert a Keys object to a string object, like this:
string key = ((char)e.Key).ToString();
Just remember that a Keys object can accept a char cast, so we can cast the resultant char object to a string object. If you want just to append it to a char object, just remove that crap, and make it like this:
char key = (char)e.key;
Solution 3
You could use a function like this:
public static string KeycodeToChar(int keyCode)
{
Keys key = (Keys)keyCode;
switch (key)
{
case Keys.Add:
return "+";
case Keys.Subtract:
return "-"; //etc...
default:
return key.ToString();
}
}
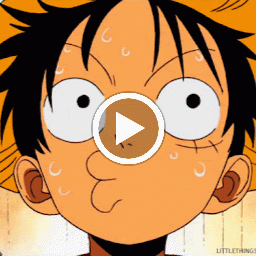
Author by
Renan Rodrigues
Updated on June 20, 2022Comments
-
Renan Rodrigues almost 2 years
How can I convert a Key (Key in KeyEventArgs) to a string.
For example, if the user enter "-" :
e.Key.ToString() = "Subtract" new KeyConverter().ConvertToString(e.Key) = "Subtract"
What I want is to get "-" for result, not "Substract"...
-
Admin almost 13 yearsVery interesting article. Do you know if this technique can be applied to an existing type?
-
svick almost 13 yearsWhy not use
Dictionary<Key, string>
? -
Ry- almost 13 years@svick: I was making that change while you said it :)
-
IAbstract almost 13 yearsThis is probably better than a
switch-case
... I think I'd get shot for suggesting aDictionary<Key, string>
-
IAbstract almost 13 years@Svick: I have suggested using an enum as the TKey before and I was told "bad idea" by several people ... although I didn't understand why it would be
-
boca almost 13 yearsDefinitely. You just have to have access tp the source to add the Description attribute
-
Admin almost 13 yearsAhh. This enumeration is part of .NET though :(
-
svick almost 13 years@IAbstract, maybe that's because in some cases, ordinary class with static properties (like
Key.Subtract
) instead of enum members and instance properties (likeKey.Char
) instead of what you want to put in the array would be better. But that's not possible here, since we're not in a position to changeKey
. -
Renan Rodrigues almost 13 yearsHow can I handle special stated key. Like RightShift + 9?
-
Ry- almost 13 years@Melursus: Check for
(e.ModifierKey & Keys.RightShift) == Keys.RightShift && e.KeyCode == Keys.D9
. -
0xDEADBEEF almost 9 yearsYou can, but it won´t help. A bijective mapping between Key-Enum value and character set value is simply not possible, due to collisions (Key.NumPad0 and Key.D0 have to have different values, but should both result in character '0'). In fact, the entire mapping of the Key-Enum is different to any character set. Just press F12 in Visual Studio on the Key-Enum and convince yourself
-
osos95 almost 9 yearsHe wanted to get the value to the Keys object as a string, not the opposite, so I can see no problem here @0xDEADBEEF