Keypress event on nested content editable (jQuery)
Solution 1
The exact code you posted in your question seems to work just fine at http://jsfiddle.net/gaby/TwgkC/3/
Tested and working with FF, Opera, Chrome, Safari, IE8 ..
only change is the removal of the comment which in its current form creates a syntax error.
The #someid
need to have focus in order for the keypress to work.
If you want your code to give focus to the element right after creating it, use the .focus()
method.
function AppendSpan()
{
$('#mydiv').append('<span id="someid" contenteditable="true">Some TExt</span>');
//Then I want to handle the keypress event on the inserted span
$('#someid').keypress(function(event){
//do something here
alert(this.id);
}).focus();// bring focus to the element once you append it..
}
Update
Two ways to handle this (the fact that there are nested contenteditable
elements), not sure if any is acceptable for your case but here they are..
- wrap the new
contenteditable
span in another one, which is set to havecontenteditable="false"
(demo: http://jsfiddle.net/gaby/TwgkC/10/) - make
#mydiv
to not becontenteditable
once you add the span..
(demo: http://jsfiddle.net/gaby/TwgkC/11/)
Solution 2
You might be better to bind the keypress event to the #mydiv element like this:
$('#mydiv').delegate("span", "keypress", function(){
console.alert('A key has been pressed: ' + this.id);
});
On further investigation though, it seems that DOM elements such as regular spans and divs are incapable of receiving focus. You may be able to get around this however, by adding a tabindex attribute to each span.
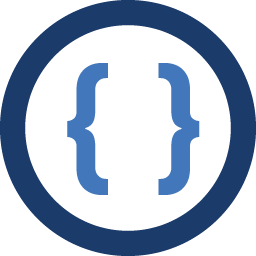
Admin
Updated on August 17, 2022Comments
-
Admin over 1 year
I have a CONTENTEDITABLE div and inside that div I have a CONTENTEDITABLE span, what I want to do is being able to handle the onkeypress event on the inner SPAN.
So, the javascript code would be:
$(function() { $('#someid').keypress(function(event){alert('test');}); });
And the HTML content would be:
<div id="mydiv" contenteditable="true"> editable follows:<span id="someid" contenteditable="true">Some TEXT</span> </div>
If you test it on a browser you'll see you won't see the 'test' dialog when you press a key over Some TEXT, I know the problem is that the event is being triggered in the parent div, so the SPAN doesn't get the event, also because it doesn't have the focus. So I'd like your help to find a solution for this.