Laravel 4 eloquent pivot table
You first have to include 'equipable' on your pivot call:
return $this->belongsToMany('User', 'user_items')
->withPivot('equipable');
Then you can ask Eloquent if your item is equipable or not:
$item = User::with('items')->get()->find(1)->items->find(2)->pivot->equipable;
Keep two things in mind:
- Eloquent uses 'items' as a key internally, so that might interfere.
- Whatever method you put before "get()" is part of the db query. Everything after "get()" is handled by PHP on the Object. The latter will be slower in most cases.
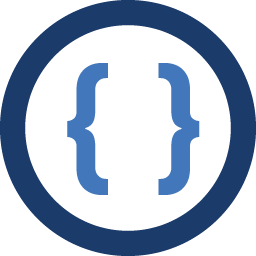
Admin
Updated on June 24, 2022Comments
-
Admin almost 2 years
I have three tables: users, items and user_items. A user has many items and a item belongs to many users.
The tables:
Users id username password Items id name equipable User_items id user_id item_id equipped
The models:
class User extends Eloquent { public function items() { return $this->belongsToMany('Item', 'user_items') ->withPivot('equipped'); } } class Item extends Eloquent { public function users() { return $this->belongsToMany('User', 'user_items'); } }
In the pivot (user_items) table I've a very important column named "equipped".
I've a form where users can equip, unequip and throw items. This form has a hidden field with the pivot (user_items) table row id. So, when a user tries to equip an item, the system checks if the item is equipable.
So, I want a object with the pivot data and the item data, based on item_id from the pivot table, I can send to the handler (where all logic is handled).
So what I've to do is to first access the pivot table and then access the item table.
Something like this (does not work):
$item = User::find(1)->items->pivot->find(1);
Is this possible to do?
-
Marko Aleksić over 10 yearsThank you! This helped me - after 2 hours banging my head from the desk.
-
Loonb about 10 yearsfor more details refer to laravel.com/docs/eloquent#working-with-pivot-tables
-
rofavadeka about 10 years:) Yay! took me a while to realize that i forgot "->pivot". +1
-
Ayobami Opeyemi about 10 yearsthis saves me from creating a model for my pivot table