Laravel 5 and Entrust. How to save user and attach role at the same time
Solution 1
I think you have this problem because you never save in the DB. Try something like this.
$role = Role::where('name','=','admin')->first();
$user = new User();
$user->name = Input::get('name');
$user->email = Input::get('email');
$user->password = Hash::make(Input::get('password'));
$user->save()
$user->attachRole($role);
return redirect('dashboard/users')->with('success-message','New user has been added');
Of course this method will work only if you auto-increment your model's id using laravel's auto-increment feature. If you are using something like uuids to uniquely identify your fields, you should also include public $incrementing = false;
inside your Model.
Make sure you include the HasRole trait inside your model.
Also take a look at Akarun's answer as it will also work.The create method, create's a new instance and also save to db so you don't need to $user->save()
Solution 2
I also use "Entrust" for managing my user permissions, but I use create
syntax to store my User. Then I use "roles()->attach", like this :
$user = User::create($this->userInputs($request));
$user->roles()->attach($request->input('role'));
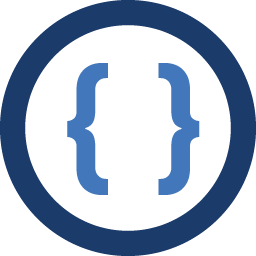
Admin
Updated on July 17, 2022Comments
-
Admin almost 2 years
Has anyone tried Entrust for User Roles & Permissions in Laravel 5?
I want to add and save user and attach role into it at the same time. here's my code
$role = Role::where('name','=','admin')->first(); $user = new User(); $user->name = Input::get('name'); $user->email = Input::get('email'); $user->password = Hash::make(Input::get('password')); if($user->save()){ $user->attachRole($role); return redirect('dashboard/users')->with('success-message','New user has been added'); }
But
$user->attachRole($role);
won't work though it works on mydatabaseSeeder
but not on my UserController.