laravel 5 custom 404
Solution 1
Go to resources/views/errors and create a 404.blade.php file with what you want on your 404 page and Laravel takes care of the rest.
Solution 2
if you want to have some global solution, you can do changes in /app/Exceptions/Handler.php by adding code bellow
public function render($request, Exception $e)
{
if ($this->isHttpException($e)) {
$statusCode = $e->getStatusCode();
switch ($statusCode) {
case '404':
return response()->view('layouts/index', [
'content' => view('errors/404')
]);
}
}
return parent::render($request, $e);
}
Solution 3
In Laravel 5 you could simply put a custom 404.blade.php under resources/views/errors and that's it. For other errors like 500 you could try the following in your app/Exeptions/Handler.php:
public function render($request, Exception $e)
{
if ( ! config('app.debug') && ! $this->isHttpException($e)) {
return response()->view('errors.500');
}
return parent::render($request, $e);
}
And do the same for 500 HTTP Exeptions
Solution 4
I like the case statement approach but it has some issues going levels deep.
However, this catches all errors:
Route::any('/{page?}',function(){
return View::make('errors.404');
})->where('page','.*');
Solution 5
Laravel 5 already has a pre-defined render method(line 43) under app/Exceptions/Handler.php. Simply insert the redirection code before parent::render. Like so,
public function render($request, Exception $e)
{
if ($e instanceof ModelNotFoundException)
{
$e = new NotFoundHttpException($e->getMessage(), $e);
}
//insert this snippet
if ($this->isHttpException($e))
{
$statusCode = $e->getStatusCode();
switch ($statusCode)
{
case '404': return response()->view('error', array(), 404);
}
}
return parent::render($request, $e);
}
Note: My view is under resources/views. You can somehow put it anywhere else you want.
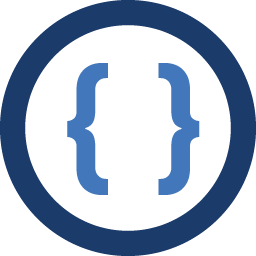
Admin
Updated on September 23, 2021Comments
-
Admin over 2 years
This is driving me crazy. I'm working with Laravel 5 and it appears that the docs for 4.2 and generating 404 pages does not work.
First, there is no global.php so I tried putting the following in routes.php:
App::missing(function($exception) { return Response::view('errors.missing', array(), 404); });
This results in an error "method missing() not found"
Debug is set to false.
I've searched and searched but so far have found no information on setting 404 pages in Laravel 5. Would appreciate any help.
-
Hayk Aghabekyan almost 9 yearsthis will work, but this is not something fully controlled by developer, as you can't use your error view inside your layout. You can see my answer.
-
enchance almost 9 yearsThis is the right answer. If you're using Twig or some other templating engine other than Blade then this allows you to load it like a normal view. Although, I must ask what is
layouts/index
? I named both toerrors.404
and it worked. -
Hayk Aghabekyan almost 9 yearslayouts is my folder for keeping main template files in it, such as index, which is my default template file.
-
manix over 8 years@HaykAghabekyan, your answers is not appropiate, because you are editing core files. This answer is the correct because you can use the error view inside of layout using
@extends()
tag. -
enchance about 8 yearsThis is the correct answer. You can even use Twig if you want more control using
404.twig
and L5 does the rest. Since it's twig, feel free to extend any template you want. -
Dovis almost 8 yearsYes, you can use
@extends()
, but how do you set variables which are used in parent view? e.g. you have header and footer with menus. -
NaturalBornCamper almost 5 years$exception->getMessage() doesn't return the "File not found" error you see on the Laravel 404 page however, so you can't get the exception message from Laravel, that's a bit annoying as it works for others
-
AlbinoDrought over 3 yearsNote that
parent::render($request, $e)
transforms some exceptions (ModelNotFoundException
) intoHttpException
s. These will not be handled by the override added here. -
Tayyab Hayat almost 3 yearsBest solution, also works with Laravel-8,