Laravel: Addition to an existing field data in a column
13,271
Solution 1
You can just retrieve the model and increment it:
$model = Some_Model::find( $id );
$model->value += 1000;
$model->save();
Solution 2
The ideal way to do this is to use the in-built Laravel function increment
$model = Some_Model::find( $id );
$model->increment('value',1000);
or
Some_Model::where('id',1)->increment('value',1000);
The documentation for the same is at http://laravel.com/docs/queries#raw-expressions
Solution 3
Using Eloquent you can write your queries as follow:
SomeTable::where('id', 1)
->update(array('value', DB::raw('value + 1000')));
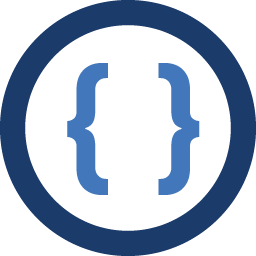
Author by
Admin
Updated on June 19, 2022Comments
-
Admin almost 2 years
Please how do i express this php mysql update code in eloquent
mysql_query("UPDATE `some_table` SET `value` = `value` + 1000 WHERE `id` = 1");
or
mysql_query("UPDATE `some_table` SET `value` = `value` + $formdata WHERE `id` = 1");
-
Admin over 10 yearsHere is my update code
Inventorie::update($arrinput['id'][$i], array( 'quantity'=> 'quantity + ' $arrinput['q'][$i], 'cprice'=>$arrinput['c'][$i], 'sprice'=>$arrinput['s'][$i] )); echo "updated"."<br>";
-
Admin over 10 yearsHere is my update code
Inventorie::update($arrinput['id'][$i], array( 'quantity'=> 'quantity + ' $arrinput['q'][$i], 'cprice'=>$arrinput['c'][$i], 'sprice'=>$arrinput['s'][$i] )); echo "updated"."<br>";
-
kodepup over 10 yearsYou left out the
DB::raw()
part of the update. That's important, since otherwise it will see 'quantity' as a string and not a column name. You want it to be'quantity'=>DB::raw('quantity + ' . $arrinput['q'][$i])
-
Admin over 10 yearsOMG! you the best! it works like magic!!!, thanks so much for the help!!!, can i do other arithmetic functions with the same method?
-
kodepup over 10 years
DB::raw()
will tell it to pass the contents to the database engine directly. You can read up on it under Query Builder at laravel.com/docs