Laravel check for unique rule without itself in update
Solution 1
This forces to ignore specific id:
'email' => 'unique:table,email_column_to_check,id_to_ignore'
replace segments table
, email_column_to_check
, id_to_ignore
in above example
You could check it here http://laravel.com/docs/4.2/validation#rule-unique
Solution 2
For those using Laravel 5 and Form Requests, you may get the id of the model of the Route-Model Binding directly as a property of the Form Request as $this->name_of_the_model->id
and then use it to ignore it from the unique rule.
For instance, if you wanted to have unique emails, but also allow an administrator to edit users, you could do:
Route:
Route::patch('users/{user}', 'UserController@update');
Controller:
public function update(UserRequest $request, User $user)
{
// ...
}
Form Request:
class UserRequest extends FormRequest
{
// ...
public function rules()
{
return [
'name' => 'required|string',
'email' => [
'required',
'email',
Rule::unique('users')->ignore($this->user()->id, 'id')
],
//...
];
}
//...
}
Please note that we are ignoring the user that is being edited, which in this case could be different than the authenticated user.
Solution 3
$request->validate([
'email' => 'unique:table_name,email,' . $user->id
]);
Solution 4
I am using Laravel 5.2
if your primary key is named 'id' table name is users_table and I want to update user_name so I do it this way
'user_name' => 'required|unique:users_table,user_name,'.$id
if your primary key is not named 'id' In my case my primary key is user_id and table name is users_table and I want to update user_name
so I do it this way
'user_name' => 'required|unique:users_table,user_name,'.$id.',user_id'
Solution 5
Try this
'email' => 'unique:table_name,column_name,'.$this->id.',id'
$this->id
will be the value from your request
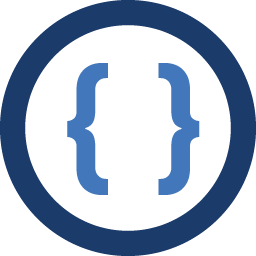
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am having my validation rules for unique in the update section.
In inserting or adding the unique rule is
'Email' => array('unique:driver_details'),
and it will check for the unique coloumn.But this fails for the update section. While updating it satisfies the other rules and in the unique of email it is checking itself for the unique coloumn and it fails but seeing its own field.
So, how can i check for the unique except its own value in the database ?
-
patricus over 9 yearspossible duplicate of Laravel 4 validation email unique constraint
-
Admin over 9 years@patricus: I got your question, but in your answer you didn't say about how to get the $id, it will be ok if they do validate for their own profile they can get their id by
Auth::user()->id
and in case of admin doing those part to others parts, should i set session and get inside model ,,, ??? -
patricus over 9 yearsHow are you currently getting the id of the record you are trying to update?
-
Admin over 9 yearsi am sending all the values to the model,
$validation = Validator::make($DriverData, DriverModel::$updaterules);
-
Admin over 9 yearsI have posted a new question here, which would be clear, can you have a look at here ,, stackoverflow.com/questions/28205762/…
-
-
Admin over 9 yearsBut How can i get the
id_to_ignore
inside the model, I can't get theAuth::user()->id
because this is admin part and doing validation for other users.. -
Uzair A. over 5 yearsWelcome to StackOverflow! Kindly consider adding some explanation with your code as well, so that OP and other users may gain insight as to how and why your answer is applicable. :)
-
Jelly Bean almost 5 yearsyou can get it from the request like this :
request()->route('route wildcard')->id
-
Daniel Cheung over 4 years
Rule::unique('users')->ignoreModel($this->user)
may also be used. -
Erenor Paz over 3 yearsJust to add a note to visitors, this method worked on Laravel/Lumen 7.0 :-)
-
totymedli over 2 yearsIf you defined your route like this:
Route::apiresource('car', 'Api\CarController')
then you can retrieve the resource's id like this:request()->route('car')
orrequest()->route()->parameters['car]
. If you are inside a request object you can use$this
instead ofrequest()
as well. -
senty about 2 years
Rule::unique('users')->ignoreModel($this->user())
is the correct syntax as of Laravel 9