Laravel faker generate gender from name
Solution 1
Looking at the documentation and an issue raised on the their Github issues section, your solution seems to be the best. Some methods allow you to specify the gender for a name so you could do like this:
$gender = $faker->randomElement(['male', 'female']);
return [
'name' => $faker->name($gender),
'email' => $faker->safeEmail,
'username' => $faker->userName,
'phone' => $faker->phoneNumber,
'gender' => $gender,
'address' => $faker->address,
'dob' => $faker->date($format = 'Y-m-d', $max = 'now'),
'password' => bcrypt('secret')
];
Hopefully this fits your requirement.
Solution 2
The method randomElements is gonna return an array with one single element, so if you want to get 'female' or 'male', don't forget to add at the end of the first line this: [0]. You need the first element (index 0) of the resulting array (that only has one element).
$gender = $faker->randomElements(['male', 'female'])[0];
One more thing. In order to obtain exactly what you want, you need to use firstName instead of name. This way the first name will be according to the gender. Do it this way:
return [ 'name' => $faker->firstName($gender), 'email' => $faker->safeEmail, 'username' => $faker->userName, 'phone' => $faker->phoneNumber, 'gender' => $gender, 'address' => $faker->address, 'dob' => $faker->date($format = 'Y-m-d', $max = 'now'), 'password' => bcrypt('secret') ];
One last thing: If you use 'Male' and 'Female', instead of 'male' and 'female', this is NOT gonna work!!
Solution 3
to do it without additional variable, do it like this
return [
'gender' => $faker->randomElements(['male', 'female']),
'name' => $faker->name(function (array $user) {return $user['gender'];})
]
hope it helps
Related videos on Youtube
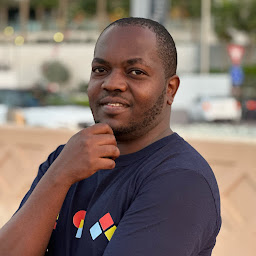
Comments
-
Morris Mukiri over 3 years
What is the optimum way of generating gender using faker, having generated a name so that the gender matches the name
return [ 'name' => $faker->name, 'email' => $faker->safeEmail, 'username' => $faker->userName, 'phone' => $faker->phoneNumber, 'gender' => $faker->randomElement(['male', 'female']),//the gender does not match the name as it is. 'address' => $faker->address, 'dob' => $faker->date($format = 'Y-m-d', $max = 'now'), 'password' => bcrypt('secret') ];
-
Marco Rivadeneyra over 5 yearsIf that's the case better use getRandomElement to get only one element instead of an array