LateError (LateInitializationError: Field 'customTypeSchemas' has not been initialized.) Amplify Plugins Error
Solution 1
You could try his
@override
List<ModelSchema> customTypeSchemas = [];
Make sure you initialize an empty list in your ModelProviderClass
Solution 2
I recently observed that error after upgrading to flutter 2.10
I was able to fix it by adding the below override method to my model provider class
@override
List<ModelSchema> customTypeSchemas = [];
After adding that, your model should look like the one below
/*
* Copyright 2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
// NOTE: This file is generated and may not follow lint rules defined in your app
// Generated files can be excluded from analysis in analysis_options.yaml
// For more info, see: https://dart.dev/guides/language/analysis-options#excluding-code-from-analysis
// ignore_for_file: public_member_api_docs, file_names, unnecessary_new, prefer_if_null_operators, prefer_const_constructors, slash_for_doc_comments, annotate_overrides, non_constant_identifier_names, unnecessary_string_interpolations, prefer_adjacent_string_concatenation, unnecessary_const, dead_code
import 'package:amplify_datastore_plugin_interface/amplify_datastore_plugin_interface.dart';
import 'Todo.dart';
export 'Todo.dart';
class ModelProvider implements ModelProviderInterface {
@override
String version = "d960b875068d46ff04d68879d96cc042";
@override
List<ModelSchema> modelSchemas = [Todo.schema];
static final ModelProvider _instance = ModelProvider();
static ModelProvider get instance => _instance;
ModelType getModelTypeByModelName(String modelName) {
switch (modelName) {
case "Todo":
{
return Todo.classType;
}
break;
default:
{
throw Exception(
"Failed to find model in model provider for model name: " +
modelName);
}
}
}
@override
List<ModelSchema> customTypeSchemas = [];
}
Solution 3
_configureAmplify
is a future method, use FutureBuilder
. You can also make it nullable and do a null check while using the model.
You can check When should I use a FutureBuilder?
Example code: check above link.
FutureBuilder(
future: _configureAmplify(), // async work
builder: (BuildContext context, snapshot) {
switch (snapshot.connectionState) {
case ConnectionState.waiting: return Text('Loading....');
default:
if (snapshot.hasError)
return Text('Error: ${snapshot.error}');
else
return Text('Result:}');
}
},
)
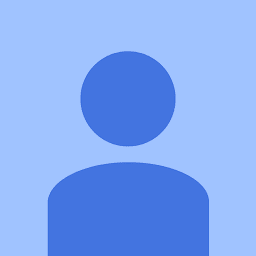
Zaid Soomro
Updated on December 01, 2022Comments
-
Zaid Soomro over 1 year
I am trying to connect Amplify Api to my project and keep getting an error that reads
LateError (LateInitializationError: Field 'customTypeSchemas' has not been initialized.)
(Screenshot of the error is provided after the code snippet) which I take to mean that in the code that Amplify provided me there is a line somewhere that haslate
prefix forcustomTypeSchemas
Field, which I can't find.This is where I am initializing Amplify.
@override void initState() { super.initState(); _configureAmplify(); } void _configureAmplify() async { if (!Amplify.isConfigured) { await Amplify.addPlugins([ AmplifyDataStore(modelProvider: ModelProvider.instance), AmplifyAPI(), ]); try { await Amplify.configure(amplifyconfig); } catch (e) { print(e.toString()); } } }
Here is my
pubspec.yaml
dependenciesdependencies: amplify_api: ^0.3.2 amplify_datastore: ^0.3.2 amplify_flutter: ^0.3.2 bloc: ^8.0.2 cupertino_icons: ^1.0.2 flutter: sdk: flutter flutter_bloc: ^8.0.1
This is what's in the
ModelProvider.dart
that Amplify downloaded./* * Copyright 2020 Amazon.com, Inc. or its affiliates. All Rights Reserved. * * Licensed under the Apache License, Version 2.0 (the "License"). * You may not use this file except in compliance with the License. * A copy of the License is located at * * http://aws.amazon.com/apache2.0 * * or in the "license" file accompanying this file. This file is distributed * on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either * express or implied. See the License for the specific language governing * permissions and limitations under the License. */ // ignore_for_file: public_member_api_docs import 'package:amplify_datastore_plugin_interface/amplify_datastore_plugin_interface.dart'; import 'Todo.dart'; export 'Todo.dart'; class ModelProvider extends ModelProviderInterface { @override String version = "d960b875068d46ff04d68879d96cc042"; @override List<ModelSchema> modelSchemas = [Todo.schema]; static final ModelProvider _instance = ModelProvider(); static ModelProvider get instance => _instance; @override ModelType getModelTypeByModelName(String modelName) { switch (modelName) { case "Todo": { return Todo.classType; } default: { throw Exception("Failed to find model in model provider for model name: " + modelName); } } } }
I did accidentally installed
amplify_core: ^0.3.2
, which I remove and ranflutter clean
and, in ios folder,pod cache clean --all
.Steps to reproduce the problem:
- Create flutter project
- Add Amplify to your project
- Add Database plugin
- Add Api to your project
- Try adding api plugin and run the app in iOS Simulator.
I am relatively new to programming and StackOverflow, so please excuse any mistakes I may have made in this post. Let me know if you need more info or you want to try something out.
Thanks in advance.
-
Zaid Soomro over 2 yearsI am still getting the same error.
-
Yeasin Sheikh over 2 yearsWhat is current error
-
Zaid Soomro over 2 yearsSame error
LateError (LateInitializationError: Field 'customTypeSchemas' has not been initialized.)
-
Yeasin Sheikh over 2 yearscan you include where is
customTypeSchemas
-
Zaid Soomro over 2 yearsThat's what I am confused about because when I do a search I see a lot of file that include this variable but don't know which one is failing. I downloaded the ModelProvider from Amplify if that helps.
-
Zaid Soomro over 2 yearsI also edited the post to include
ModelProvider.dart
file -
Zaid Soomro over 2 yearsI found the file it is part of amplify_datastore_plugin_interface. Does that help?
-
Zaid Soomro about 2 yearsI just removed everything related to AWS in my project and started over again and it worked, I hadn't spent too much time on it so it wasn't that big of a deal. Thanks for you respond though.