Launch screen from native background service
On Android you need to display a high priority notification. This displays the slide-down notification panel which will appear over the lock screen or another app. Since you are using native code already, you can create this notification there, or send a message to the Dart side (as you are doing, using MethodChannel
) where it can use the flutter_local_notifications
plugin to display it. When the user click the notification, your flutter app is brought to the foreground. In Java you might use code similar to this:
// Create an intent which triggers the fullscreen notification
Intent intent = new Intent(Intent.ACTION_MAIN, null);
intent.setAction("SELECT_NOTIFICATION");
Class mainActivityClass = getMainActivityClass(context);
intent.setClass(context, mainActivityClass);
PendingIntent pendingIntent = PendingIntent.getActivity(context, 1, intent, PendingIntent.FLAG_UPDATE_CURRENT);
// Build the notification as an ongoing high priority item to ensures it will show as
// a heads up notification which slides down over top of the current content.
final Notification.Builder builder = new Notification.Builder(context, CHANNEL_ID);
builder.setOngoing(true);
// Set notification content intent to take user to fullscreen UI if user taps on the
// notification body.
builder.setContentIntent(pendingIntent);
// Set full screen intent to trigger display of the fullscreen UI when the notification
// manager deems it appropriate.
builder.setFullScreenIntent(pendingIntent, true);
// Setup notification content.
int resourceId = context.getResources().getIdentifier("app_icon", "drawable", context.getPackageName());
builder.setSmallIcon(resourceId);
builder.setContentTitle("Your notification title");
builder.setContentText("Your notification content.");
MyPlugin.notificationManager().notify(someId, builder.build());
Then, for Android 8.1 or higher, add the following to your MainActivity
class, found under the android/app/src/main/java/packageName/ folder
GeneratedPluginRegistrant.registerWith(this);
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O_MR1) {
setShowWhenLocked(true);
setTurnScreenOn(true);
}
(This shows the Flutter app even when the screen is locked.)
Flutter only has one activity, so the above code will bring that Flutter activity to the foreground (note that you don't always see the notification, but sometimes you do - if you set it to autoCancel then touching it clears it). It's up to you to build the correct screen in Flutter, which you can do as you send the notification. Use Navigator.push
or equivalent to change the page that Flutter is showing.
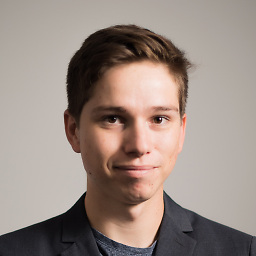
Jérémy
Updated on December 08, 2022Comments
-
Jérémy 18 days
I have a simple Flutter app that start a native background service using
MethodChannel
. This native background service notify my Flutter app usingBasicMessageChannel<String>
to display text when a particular native information is catched. All of this work perfectly when my app is in foreground. When an other app is in foreground, I can't see the text without having to switch to my app.I want that my native service can display a particular Flutter screen even if an other application is running in foreground.
It can be perceived as not user friendly, but this is an message of utmost importance.
Any suggestion or solution will be welcome !
Note : Native service is for the moment only available in Java for Android, I'm working on C# for IOS side.
-
Jérémy almost 4 yearsThank's Richard, but I don't want to display a normal notification, and on a user action, open an activity. I want to display an activity in foreground without asking any user action. Or if it's possible a full screen notification.
-
Richard Heap almost 4 yearsThe above (edited) code definitely works, so try it. It does show a notification as well as bringing the Flutter app to the front, but in my app I want that for the sound. You might be able to fine-tune it for your application.
-
Jérémy almost 4 yearsMy bad, everything's perfect !
-
ali almost 4 years@Jérémy Can i see on how you do it on the dart file?
-
Marco Alves almost 3 years@RichardHeap I'm very new to flutter, how to implement it? What do I need to do in order to implement it?
-
Savad over 2 years@RichardHeap I am looking for a solution for a long time . can i see how you implemented this in flutter (Code) .Thank you