Length of string WITHOUT spaces (C#)
Solution 1
This returns the number of non-whitespace characters:
"I am Bob".Count(c => !Char.IsWhiteSpace(c));
White space characters are the following Unicode characters:
- Members of the SpaceSeparator category, which includes the characters SPACE (U+0020), OGHAM SPACE MARK (U+1680), MONGOLIAN VOWEL SEPARATOR (U+180E), EN QUAD (U+2000), EM QUAD (U+2001), EN SPACE (U+2002), EM SPACE (U+2003), THREE-PER-EM SPACE (U+2004), FOUR-PER-EM SPACE (U+2005), SIX-PER-EM SPACE (U+2006), FIGURE SPACE (U+2007), PUNCTUATION SPACE (U+2008), THIN SPACE (U+2009), HAIR SPACE (U+200A), NARROW NO-BREAK SPACE (U+202F), MEDIUM MATHEMATICAL SPACE (U+205F), and IDEOGRAPHIC SPACE (U+3000).
- Members of the LineSeparator category, which consists solely of the LINE SEPARATOR character (U+2028).
- Members of the ParagraphSeparator category, which consists solely of the PARAGRAPH SEPARATOR character (U+2029).
- The characters CHARACTER TABULATION (U+0009), LINE FEED (U+000A), LINE TABULATION (U+000B), FORM FEED (U+000C), CARRIAGE RETURN (U+000D), NEXT LINE (U+0085), and NO-BREAK SPACE (U+00A0).
Solution 2
No. It doesn't.
string s = "I am Bob";
Console.WriteLine(s.Replace(" ", "").Length); // 6
Console.WriteLine(s.Replace(" ", null).Length); //6
Console.WriteLine(s.Replace(" ", string.Empty).Length); //6
Here is a DEMO
.
But what are whitespace characters?
http://en.wikipedia.org/wiki/Whitespace_character
Solution 3
You probably forgot to reassign the result of Replace
. Try this:
string s = "I am bob";
Console.WriteLine(s.Length); // 8
s = s.Replace(" ", "");
Console.WriteLine(s.Length); // 6
Solution 4
A pretty simple way is to write an extension method that will do just that- count the characters without the white spaces. Here's the code:
public static class MyExtension
{
public static int CharCountWithoutSpaces(this string str)
{
string[] arr = str.Split(' ');
string allChars = "";
foreach (string s in arr)
{
allChars += s;
}
int length = allChars.Length;
return length;
}
}
To execute, simply call the method on the string:
string yourString = "I am Bob";
int count = yourString.CharCountWithoutSpaces();
Console.WriteLine(count); //=6
Alternatively, you can split the string an way you want if you don't want to include say, periods or commas:
string[] arr = str.Split('.');
or:
string[] arr = str.Split(',');
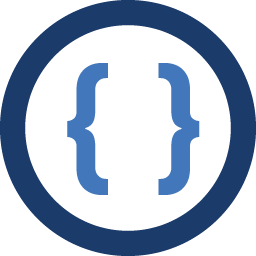
Admin
Updated on June 12, 2022Comments
-
Admin almost 2 years
Quick little question...
I need to count the length of a string, but WITHOUT the spaces inside of it. E.g. for a string like "I am Bob",
string.Length
would return 8 (6 letters + 2 spaces).I need a method, or something, to give me the length (or number of) just the letters (6 in the case of "I am Bob")
I have tried the following
s.Replace (" ", ""); s.Replace (" ", null); s.Replace (" ", string.empty);
to try and get "IamBob", which I did, but it didn't solve my problem because it still counted "" as a character.
Any help?
-
AdrianHHH about 11 yearsHow fast is
string.Lenght
(or evenstring.Length
)? Would it be better to assign it to a variable before the loop and then use that variable twice? And why all the@
characters in@string
? -
Jeppe Stig Nielsen about 11 yearsOr maybe
"I am Bob".Length - "I am Bob".Count(Char.IsWhiteSpace)
which does a subtraction but doesn't need to create a lambda. No, I am probably micro-optimizing. -
Jeppe Stig Nielsen about 11 yearsGood point he probably forgot to re-assign. I agree. Otherwise it would have worked for him. But there is no reason to have multiple
Replace
after each other like you have above. Once all spaces are replaced by""
, there's no more spaces to replace in the calls that follow. -
burning_LEGION about 11 years@AdrianHHH this is template to create method therefore i don't optimize it, read about c# to know what is
@
-
Jeppe Stig Nielsen about 11 yearsGreat, maybe this comment will get +1 as well :-) If your string
"I am Bob"
might contain combining accents or surrogate pairs (Unicode characters that need two 16-bit values in UTF-16), then the interpretation of the count (or evenLength
) should be careful. -
Admin about 11 yearsWow, now I feel incredibly stupid... Exactly right, I didn't assign the new value so it just got lost. Made a local variable to hold the new string without spaces, works like a charm. THANK YOU!