Limit in For-Each Loop
Solution 1
If you must you an enhanced for loop, you must introduce your own counter in order to test the number of iterations :
int limit = 0;
for (XtfPing ping : xtf.getPings())
{
if (limit <= 10000) {
// do something
} else {
// do something else
}
limit++;
}
An alternative (if getPings()
returns a Collection that supports random access (such as List) or an array) is to replace your enhanced for loop with a traditional for loop, in which the iteration number is built in.
For example, if getPings
returns a List
:
for (int i = 0; i < xtf.getPings().size(); i++)
{
XtfPing ping = xtf.getPings().get(i);
if (i <= 10000) {
// do something
} else {
// do something else
}
}
Solution 2
I know this doesn't fit exactly the OP's situation, but maybe this will help someone else looking to implement an iterate limit using Java 8:
List<Object> myList = ...
List<Object> first10000 = myList.stream().limit(10000).collect(Collectors.toList());
for (Object obj : first10000) {
// do stuff
}
or more simply:
List<Object> myList = ...
myList.stream().limit(1000).forEach(o -> {
// do stuff
});
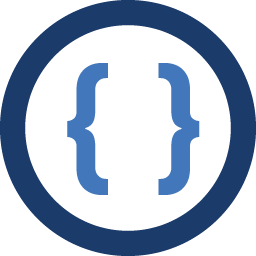
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm a beginner in Java and a geomatics student.
I work with an XTF image, its works like TIFF. This image store about 20000 lines called pings with several informations : coordinates, start time, stop time... The treatments I use on Intellij become too heavy but it works well. I want to cut in two the informations stored in my XTF image : 1 image with the 10000 first pings and the other with the 20000 last pings. Later I would gather the two images. My question is simple : how, with a “for each” loop can I order a limit (<=10000) ? I stored the information in a csv file.
for (XtfPing ping : xtf.getPings()) { writer.write( Double.toString( ping.x) ); writer.write( "," ); writer.write( Double.toString( ping.y) ); writer.write( "\n" ); } writer.close();
-
Admin almost 9 yearsThank you ! It works very well and it's very simple ! :)
-
Admin almost 9 yearsWith the second solution, I have an exception : java.lang.IndexOutOfBoundsException: Index: 19101, Size: 19101
-
Eran almost 9 years@Manimalis Perhaps you wrote
<=
instead of<
in your loop. -
byInduction over 4 yearsThis should be the accepted answer if OP can utilize stream
-
Amir Dora. almost 3 yearsThis is proper way to do it, exactly what I was looking for.