Limit max width of Container in Flutter
Solution 1
You can add a constraint to the Container Widget with the preferred maxWidth
like this:
Widget build(context) {
return Row(
mainAxisSize: MainAxisSize.min,
children: [
Container(
constraints: BoxConstraints(minWidth: 100, maxWidth: 200),
padding: EdgeInsets.all(10),
decoration: BoxDecoration(
color: color ?? Colors.blue,
borderRadius: BorderRadius.circular(10)
),
child: msg
)
],
);
}
I hope this helps!
Solution 2
Use ConstrainedBox
with BoxConstraints
maxWidth
, wrapped in a Flexible()
widget. The Flexible widget allows for the box to resize for smaller screens as well.
Flexible(
child: ConstrainedBox(
constraints: BoxConstraints(maxWidth: 150),
child: Container(
color : Colors.blue,
child: Text('Your Text here'),
),
),
),
Solution 3
This required to be inside Row
or Column
Widget
1. Row
Row(
children: [
Container(
constraints: BoxConstraints(maxWidth: 300),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(8.0)),
child: Text("Long Text....")
),
],
);
2. Column
Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Container(
constraints: BoxConstraints(maxWidth: 300),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(8.0)),
child: Text("Long Text....")
),
],
);
Here in both examples, it's working because Column
and Row
allow it's children to expand to it's given constraint/size.
Note: If a child wants a different size from its parent and the parent doesn’t have enough information to align it, then the child’s size might be ignored.
Solution 4
For me at least putting the Constrained box in IntrinsicWidth has solved issue.
IntrinsicWidth(
child: Container(
constraints: BoxConstraints(maxWidth: 200),
child: Row(
children: [
],
),
),
);
Solution 5
Use Constraints
property in Container
widget.
use maxWidth
& minWidth
value to achieve the requirement.
Container(
constraints: BoxConstraints(minWidth: 150, maxWidth: 300),
padding: EdgeInsets.all(10),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(5)),
child: Text("")
)
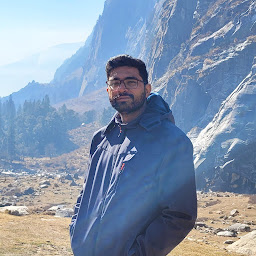
Nishant Desai
Updated on September 13, 2021Comments
-
Nishant Desai over 2 years
Widget build(context) { return Row( mainAxisSize: MainAxisSize.min, children: [ Container( width: 300, padding: EdgeInsets.all(10), decoration: BoxDecoration( color: color ?? Colors.blue, borderRadius: BorderRadius.circular(10) ), child: msg ) ], ); }
This is build method of my widget and It renders this UI depending on what I pass as
msg
paramterNow the issue I am facing is that I am not able to wrap the text inside this container/blue box without setting it's width but If I set width of container/blue box then it will always stay that wide no matter how short the text is.
Now is there a way to set maxWidth (like let's say 500) of container/blue box? So that the blue box will become as wide as required for small text like "Loading" and for long text it will expand till it reaches width of 500 units and then will wrap the text ?
Required output for long text:
For small text like loading I dont want any change in UI but for long text I want it to look like this.
-
Nishant Desai about 5 yearsI don't want to give fixed size to the container because container is the one which is providing the blue background and if I give it a fixed size then even when I show "Loading" message the blue container will not shrink to adapt to short text.
-
Roc Boronat over 3 yearsI just tried it, and the problem is that it overflows the screen in case the screen is not that large.
-
Roc Boronat over 3 yearsFixed! Just put this ConstrainerBox inside a Flexible :·)
-
Matias de Andrea about 2 yearsIs recommend by Flutter, avoid use IntrinsicWidth and IntrinsicHeight when posible, because is too expensive to build