Line 0: Parsing error: Cannot read property 'map' of undefined
Solution 1
Is this coming from eslint-typescript? If so, check that your version of typescript is not a dev/nightly build.
Solution 2
Edit: as noted by Meng-Yuan Huang, this issue no longer occurs in react-scripts@^4.0.1
This error occurs because react-scripts
has a direct dependency on the 2.xx range of @typescript-eslint/parser
and @typescript-eslint/eslint-plugin
.
You can fix this by adding a resolutions field to your package.json
as follows:
"resolutions": {
"**/@typescript-eslint/eslint-plugin": "^4.1.1",
"**/@typescript-eslint/parser": "^4.1.1"
}
NPM users: add the resolutions field above to your package.json
but use npx npm-force-resolutions to update package versions in package-lock.json
.
Yarn users: you don't need to do anything else. See selective dependency resolutions for more info.
NOTE: if you're using a monorepo/Yarn workspaces, the resolutions
field must be in the top-level package.json
.
NOTE: yarn add
and yarn upgrade-interactive
don't respect the resolutions
field and they can generate a yarn.lock
file with incorrect versions as a result. Watch out.
Solution 3
For future Googlers:
I had the same issue just now on TypeScript 4.0.2 in a Vue.js 2 project. I fixed it by upgrading @typescript-eslint/eslint-plugin
and @typescript-eslint/parser
to the latest that npm would give me using @latest
, which at the time was 3.3.0 and 3.10.1, respectively.
Solution 4
Your version of TypeScript is not compatible with your eslint. You can fix it by upgrading these two dependencies to the latest version.
TypeScript 4.0.5 is compatible with version 4.6.0
"devDependencies": {
"@typescript-eslint/eslint-plugin": "^4.6.0",
"@typescript-eslint/parser": "^4.6.0",
}
TypeScript 4.1.5 is compatible with version 4.18.0
"devDependencies": {
"@typescript-eslint/eslint-plugin": "^4.18.0",
"@typescript-eslint/parser": "^4.18.0",
}
TypeScript 4.2.4 is compatible with version 4.23.0
"devDependencies": {
"@typescript-eslint/eslint-plugin": "^4.23.0",
"@typescript-eslint/parser": "^4.23.0",
}
TypeScript 4.3.2 is compatible with version 4.25.0
"devDependencies": {
"@typescript-eslint/eslint-plugin": "^4.25.0",
"@typescript-eslint/parser": "^4.25.0",
}
TypeScript 4.5.5 is compatible with version 5.10.2
"devDependencies": {
"@typescript-eslint/eslint-plugin": "^5.10.2",
"@typescript-eslint/parser": "^5.10.2",
}
TypeScript 4.6.2 is compatible with version 5.15.0
"devDependencies": {
"@typescript-eslint/eslint-plugin": "^5.15.0",
"@typescript-eslint/parser": "^5.15.0",
}
TypeScript 4.7.2 is compatible with version 5.26.0
"devDependencies": {
"@typescript-eslint/eslint-plugin": "^5.26.0",
"@typescript-eslint/parser": "^5.26.0",
}
Solution 5
Try playing around with variable types inside the interfaces. E. g I've got this error when I had such state interface:
interface State{
foo: []
}
but when I've changed the type of array it worked:
interface State{
foo: string[]
}
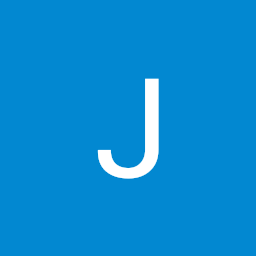
Jon Hernandez
Updated on March 21, 2022Comments
-
Jon Hernandez about 2 years
Currently starting up the server on my client side, the error above is what I have been getting. I am using Typescript, React, ESlint. I can't seem to go forward since this error has been haunting me. The github page for Eslint hasn't been much help either.
This error went up after I had created the useMutation component and exported it in the index.ts, Not sure how to get rid of this error.
Below is my package.json
{ "name": "tinyhouse_client", "version": "0.1.0", "private": true, "dependencies": { "@testing-library/jest-dom": "^4.2.4", "@testing-library/react": "^9.3.2", "@testing-library/user-event": "^7.1.2", "@types/jest": "^24.0.0", "@types/node": "^12.0.0", "@types/react": "^16.9.35", "@types/react-dom": "^16.9.0", "@typescript-eslint/parser": "^3.0.2", "react": "^16.13.1", "react-dom": "^16.13.1", "react-scripts": "3.4.1", "typescript": "~2.23.0" }, "resolutions": { "@typescript-eslint/eslint-plugin": "^2.23.0", "@typescript-eslint/parser": "^2.23.0", "@typescript-eslint/typescript-estree": "^2.23.0" }, "scripts": { "start": "react-scripts start", " build": "react-scripts build", "test": "react-scripts test", "eject": "react-scripts eject" }, "eslintConfig": { "extends": "react-app" }, "browserslist": { "production": [ ">0.2%", "not dead", "not op_mini all" ], "development": [ "last 1 chrome version", "last 1 firefox version", "last 1 safari version" ] }, **strong text** "proxy": "http://localhost:9000" }
Below is my index.ts
export * from './server'; export * from './useQuery'; export * from './useMutation';
And my useMutation.ts
import { useState } from 'react'; import { server } from './server'; interface State<TData> { data: TData | null; loading: boolean; error: boolean; } type MutationTuple<TData, TVariables> = [ (variables?: TVariables | undefined) => Promise<void>, State<TData> ]; export const useMutation = <TData = any, TVariables = any>( query: string ): MutationTuple<TData, TVariables> => { const [state, setState] = useState<State<TData>>({ data: null, loading: false, error: false, }) const fetch = async (variables?: TVariables) => { try { setState({ data: null, loading: true, error: false }); const { data, errors } = await server.fetch<TData, TVariables>({ query, variables }); if (errors && errors.length) { throw new Error(errors[0].message); } setState({ data, loading: false, error: false }); } catch (err) { setState({ data: null, loading: false, error: true }); throw console.error(err); } } return [fetch, state]; };
-
Jon Hernandez almost 4 yearsgithub.com/Jonathanh7/tinyhouse_v1, here is the link to my github repo so you can see that errors I have been getting on your Editors.
-
smac89 almost 3 yearsSimilar question here: stackoverflow.com/questions/63825685/…
-
-
Johannes Klauß over 3 yearsAlthough this won't work if you are using an unejected Create React App bootstrapped project.
-
mcartur over 3 yearsHi @JohannesKlauß ! were you able to find out how could you solve this issue with an unejected Create React App project? I am still getting this error. Thanks!
-
Johannes Klauß over 3 yearsHaven't checked yet, but you should be able to force a single version via the
resolutions
field inside the package.json classic.yarnpkg.com/en/docs/selective-version-resolutions -
mcartur over 3 yearsHi again @JohannesKlauß thank you for your response! I finally changed my typescript version to 3.9.5 and the error stopped.
-
Johannes Klauß over 3 yearsYou can also subscribe to this issue here to track the progress: github.com/facebook/create-react-app/issues/9515
-
Jose A over 3 years@JohannesKlauß Thanks for the tip, it seems that yarn resolutions don't do any justice either. It's still throwing an error, even by specifying
@typescript-eslint/eslint-plugin and @typescript-eslint/parser
to4.0.1
. Had to revert back to 3.9.7 and delete the .cache folder as others said. -
Johannes Klauß over 3 yearsI think every version < 4 should work. The vital part is the fresh install, so no cache is used.
-
mohammadreza berneti over 3 yearsIt fixed after deleting the .cache folder
-
Rob Philipp over 3 years@JohannesKlauß I also had to revert to 3.9.7 and delete my node_modules directory.
-
manroe over 3 yearsThis helped, but I ran into issues with Babel after too, so deleting the whole
node_modules
directory solved both. -
feupeu over 3 yearsI also had to remove the entire
node_modules
directory for this to work -
David Sherret over 3 yearsThis fixes the bug because
[]
is parsed as a tuple type, whilestring[]
is pared as an array type. In TS 4.0 a property was renamed on the tuple type node in the AST that breaks this. So a possible quick way around the error is to just avoid tuple types if able... -
kca over 3 years
-
ClownBaby over 3 yearsFor me this was solved by downgrading typescript to <4.0, removing
node_modules
and lock file. Something with create-react-app doesn't support ts 4 yet it seems like. -
tim.rohrer over 3 years@DavidSherret's comment and this answer were what worked for me. Updating the
eslint-plugin
andparser
had no affect. But, reworking the type definition resolve the issue. -
Zsolt Meszaros over 3 yearsIf you remove
package.json
,yarn install
won't do anything. -
mojmir.novak over 3 yearsNot sure if this is the correct answer for all cases. Regardless of versions of tslint or Typescript problem is in wrong types. Check this:
myValue: [][]
versusmyValue: string[][]
. Check answers below. -
bas over 3 yearsThank you! I also had the problem that my returned tuple type
[string, string]
gave the errorCannot read property 'map' of undefined
. This unhelpful message drove me crazy until I found this. -
Dmitry Reutov over 3 yearsno need to avoid use tuples, just write
someTuple: [v1: string, v2: string]
to make it work -
Meng-Yuan Huang over 3 yearsThanks for your information. However, I fix this problem by updating react-scripts from 3.4.4 to 4.0.1.
-
Aniket kale about 3 yearsgithub.com/typescript-eslint/typescript-eslint/issues/… This helps me to fix this issue. Thanks.
-
M-x almost 3 yearsExtremely useful, this fixed my issues!
-
M-x almost 3 yearsAlso replacing [number, number] with number[] as well as other types fixes the remaining errors.
-
alvaro.canepa almost 3 yearsSave my day! this must be the accepted answer!
-
zak almost 3 yearsvery helpful, I had similar issue, where I declared an array without specifying the type.
-
Harald over 2 yearsI could not find a resource describing the correct versions to use. In case you have one, it would be great to add the link.
-
Devin Rhode over 2 yearsSeconding comment from @Harald - I'd love to know how you derived this list!
-
Black over 2 yearsI update my dependencies and when I see it working then I update this answer
-
InterstellarX about 2 yearsThis was immensely helpful! This response should be the accepted answer.
-
Jon Hernandez about 2 yearsI made this the answer since the version I was previously using was a nightly build. Downgrading it to a none nightly build solved my issue.