Line of dots between items
13,624
Solution 1
I think you look for something like this:
html
<div>
<div>Marinated Olives</div>
<div class="dot"></div>
<div>4.00E</div>
</div>
css
.dot{
border-bottom: dotted 3px orange;
width: 100px;
float: left;
position: relative;
display: block;
height: 12px;
margin: 0 5px 0 5px;
}
div:first-child, div:last-child{
float:left;
}
You can play with width to adjust in your likes.
Also another approach using css :after
html
<div>
<div id="dotted">Marinated Olives</div>
<div>4.00E</div>
</div>
css
div{
float:left;
}
#dotted::after{
content: "..................";
letter-spacing: 4px;
font-size: 18px;
color:orange;
margin-left:20px;
}
Here you can play with content and letter-spacing. Hope it helps :)
Solution 2
.text-div {
background-image: linear-gradient(to right, #000 10%, rgba(255, 255, 255, 0) 0%);
background-position: 0 14px;
background-size: 10px 1px;
background-repeat: repeat-x;
width: 100%;
height:25px;
}
.text-span {
background: #fff;
padding-right: 10px
}
.pull-right {
float: right;
padding-left: 10px
}
<ol>
<li>
<div class="text-div">
<span class="text-span">Item:</span>
<span class="text-span pull-right">$125.00</span>
</div>
</li>
<li>
<div class="text-div">
<span class="text-span">Very long name of the item:</span>
<span class="text-span pull-right">$20.00</span>
</div>
</li>
<li>
<div class="text-div">
<span class="text-span">Not long name:</span>
<span class="text-span pull-right">$30.00</span>
</div>
</li>
</ol>
Solution 3
something like this?
ol li {
font-size: 20px
}
.dot-div {
border-bottom: thin dashed gray;
width: 100%;
height: 14px
}
.text-div {
margin-top: -14px
}
.text-span {
background: #fff;
padding-right: 5px
}
.pull-right {
float: right;
padding-left: 5px
}
<ol>
<li>
<div class="dot-div"></div>
<div class="text-div">
<span class="text-span">Item one</span>
<span class="text-span pull-right">400$</span>
</div>
</li>
<li>
<div class="dot-div"></div>
<div class="text-div">
<span class="text-span">Item two with long text</span>
<span class="text-span pull-right">400$</span>
</div>
</li>
<li>
<div class="dot-div"></div>
<div class="text-div">
<span class="text-span">Item three midium</span>
<span class="text-span pull-right">400$</span>
</div>
</li>
</ol>
Solution 4
Use a div with absolute positioning? White backgrounds for paragraphs? Valid for any length of menu-item-name. Play around with it, good luck!
<div class='item_wrapper'>
<p class='item_name'>Marinated olives</p>
<p class='item_price'>4,00€</p>
<div class='dotted_line'></div>
</div>
.item_wrapper{
width:100%;
clear: both;
}
.dotted_line{
border-top:dotted 2px orange;
position:relative;
width:100%;
top:33px;
z-index:-1;
}
p{
position:relative;
background:white;
padding:0px 10px;
}
.item_name{
float:left;
}
.item_price{
float:right;
}
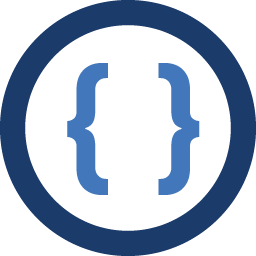
Author by
Admin
Updated on July 25, 2022Comments
-
Admin almost 2 years
Restaurant web site and menu. I need to get "line of dots" between menu item and price. I need to get it without writing dots manually one by one. This feature should work automatically.
Is it possible to create this by using background of span or div etc?
Where I am
Where I need to be
Thanks for advance.