Listview overlaps with ToolBar
Solution 1
I had a similar problem and after following the top answer, I rewrote my code to use RecyclerView
and got the same issue. I then added this behavior to my content layout and it worked
app:layout_behavior="@string/appbar_scrolling_view_behavior"
Solution 2
CoordinatorLayout
's ScrollingViewBehavior
only works with views that support nested scrolling (for example RecyclerView
). ListView
does not support it by default, so the CoordinatorLayout
is not handling the position of the ListView
correctly.
If you want all the scrolling behaviours of the design support library, you could try converting your list to a RecyclerView
. ListView
also gained nested scrolling support in API 21, which you could try to enable with setNestedScrollingEnabled(true)
, but that will obviously only work on Lollipop and newer versions.
More information here: ScrollingViewBehavior for ListView
Solution 3
I'm getting the same problem where part of my listview is overlapped by toolbar. I'm able to make it work in android 4.4.4(Kitkat) but the issue could not be resolved in android 5.1(Lolipop) So, Here is my solution to resolve this issue
layout/activity_main.xml
<include layout="@layout/app_bar_main" android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior"/>
<android.support.design.widget.NavigationView android:id="@+id/nav_view"
android:layout_width="wrap_content" android:layout_height="match_parent"
android:layout_gravity="start" android:fitsSystemWindows="true"
app:headerLayout="@layout/nav_header_main" app:menu="@menu/activity_main_drawer"
/>
The mistake which I made was that, adding the listview in activity_main.xml which we should not add instated we should add listview in app_bar_main.xml
layout/app_bar_main.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.design.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" android:fitsSystemWindows="true"
tools:context=".MainActivity">
<android.support.design.widget.AppBarLayout android:layout_height="wrap_content"
android:layout_width="match_parent" android:theme="@style/AppTheme.AppBarOverlay">
<android.support.v7.widget.Toolbar android:id="@+id/toolbar"
android:layout_width="match_parent" android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary" app:popupTheme="@style/AppTheme.PopupOverlay" />
</android.support.design.widget.AppBarLayout>
<FrameLayout
android:id="@+id/fragment_container"
android:layout_width="match_parent"
android:layout_height="match_parent">
</FrameLayout>
<ListView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/AartilistView"
android:layout_below="@+id/textView"
android:layout_alignParentStart="true"
android:layout_marginTop="?android:attr/actionBarSize"
/>
<android.support.design.widget.FloatingActionButton android:id="@+id/fab"
android:layout_width="wrap_content" android:layout_height="wrap_content"
android:layout_gravity="bottom|end" android:layout_margin="16dp"
android:src="@android:drawable/ic_dialog_email" />
</android.support.design.widget.CoordinatorLayout>
Other files containing the code is layout/nav_header_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="190dp"
android:background="@drawable/background_material_red"
android:orientation="vertical"
>
<de.hdodenhof.circleimageview.CircleImageView
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/profile_image"
android:layout_width="70dp"
android:layout_height="70dp"
android:src="@drawable/logo"
app:border_color="#FF000000"
android:layout_marginLeft="24dp"
android:layout_centerVertical="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_marginStart="24dp" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/app_name"
android:textSize="14sp"
android:textColor="#FFF"
android:textStyle="bold"
android:gravity="left"
android:paddingBottom="4dp"
android:id="@+id/username"
android:layout_above="@+id/email"
android:layout_alignLeft="@+id/profile_image"
android:layout_alignStart="@+id/profile_image" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/email"
android:id="@+id/email"
android:gravity="left"
android:layout_marginBottom="8dp"
android:textSize="14sp"
android:textColor="#fff"
android:layout_alignParentBottom="true"
android:layout_alignLeft="@+id/username"
android:layout_alignStart="@+id/username" />
</RelativeLayout>
layout/item_view.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent" android:layout_height="match_parent">
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/icon_image"
android:layout_alignParentTop="true"
android:layout_alignParentStart="true"
android:src="@drawable/img"
android:maxHeight="80dp"
android:maxWidth="80dp"
android:longClickable="false"
android:adjustViewBounds="true" android:paddingTop="10dp" android:paddingBottom="10dp" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textAppearance="?android:attr/textAppearanceLarge"
android:text="Large Description"
android:id="@+id/icon_text"
android:layout_alignBottom="@+id/icon_image"
android:layout_toEndOf="@+id/icon_image"
android:layout_alignParentEnd="false"
android:layout_alignParentStart="false"
android:paddingBottom="20dp"
android:paddingLeft="20dp" />
</RelativeLayout>
menu/activity_main_drawer.xml
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<group android:checkableBehavior="single">
<item android:id="@+id/nav_info_details" android:icon="@drawable/ic_menu_info_details"
android:title="About us" />
<item android:id="@+id/nav_rate" android:icon="@drawable/ic_menu_star"
android:title="Rate App" />
<item android:id="@+id/nav_share" android:icon="@drawable/ic_menu_share"
android:title="Share App" />
<item android:id="@+id/nav_facebook" android:icon="@drawable/ic_menu_fb"
android:title="Follow me on Facebook" />
</group>
<item android:title="Communicate">
<menu>
<item android:id="@+id/nav_whatsapp" android:icon="@drawable/ic_menu_whatsapp"
android:title="Whatsapp us to share your" />
<item android:id="@+id/nav_email" android:icon="@drawable/sym_action_email"
android:title="Tell us how you feel about App" />
</menu>
</item>
</menu>
make sure you build the project and then run it. Don't add your listview in activity_main.xml instead add it in app_bar_main.xml which you'll include in activity_main.xml anyways.
Solution 4
XML design showing unchecked "Show layout decorations":
XML design showing checked "Show layout decorations":
After checking this option, add your ListView and set default margins (Eg. 8dp). This will NOT hide the first item in list view.
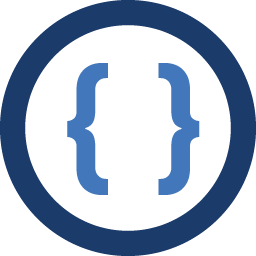
Admin
Updated on June 29, 2022Comments
-
Admin almost 2 years
I am using android design support library.
ListView
first row overlaps withToolbar
widget.In my
MainActivity
i added coordination layout and in first TAB fragment addedListView
layout.But first row of the
ListView
cut because ifToolbar
tabs.How to fix this problem?
Main.xml
<?xml version="1.0" encoding="utf-8"?> <android.support.v4.widget.DrawerLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:id="@+id/drawer_layout" android:layout_width="match_parent" android:layout_height="match_parent"> <RelativeLayout android:id="@+id/coordi" android:layout_width="match_parent" android:layout_height="match_parent"> <android.support.design.widget.CoordinatorLayout android:id="@+id/coordinatorLayout" android:layout_width="match_parent" android:layout_height="match_parent"> <android.support.design.widget.AppBarLayout android:id="@+id/appBarLayout" android:layout_width="match_parent" android:layout_height="wrap_content" android:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar"> <android.support.v7.widget.Toolbar android:id="@+id/toolbar" android:layout_width="match_parent" android:layout_height="?attr/actionBarSize" android:background="?attr/colorPrimary" app:layout_scrollFlags="scroll|enterAlways" app:popupTheme="@style/ThemeOverlay.AppCompat.Light" /> <android.support.design.widget.TabLayout android:id="@+id/tabLayout" android:layout_width="match_parent" android:layout_height="wrap_content" app:tabIndicatorColor="#FFEB3B" app:tabIndicatorHeight="6dp" app:tabSelectedTextColor="@android:color/white" app:tabTextColor="@android:color/white" /> </android.support.design.widget.AppBarLayout> <android.support.v4.view.ViewPager android:id="@+id/viewPager" android:layout_width="match_parent" android:layout_height="match_parent" /> <android.support.design.widget.FloatingActionButton android:id="@+id/fab" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="end|bottom" android:layout_margin="16dp" android:src="@drawable/plus" /> </android.support.design.widget.CoordinatorLayout> </RelativeLayout> <android.support.design.widget.NavigationView android:id="@+id/navigation_view" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_gravity="start" app:headerLayout="@layout/layout_drawer_header" /> </android.support.v4.widget.DrawerLayout> list_view.xml: ------------- <?xml version="1.0" encoding="utf-8"?> <ListView xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/listView1" android:layout_width="match_parent" android:layout_height="match_parent"></ListView>
-
Gabriele Mariotti over 8 yearsIt is not correct.
ListView
support the nested Scroll behavior with API 21+ Chech here :stackoverflow.com/questions/30696611/…. Also you should not use aListView
inside aScrollView
orNestedScrollView
. -
mankaho1987 over 7 yearsSorry Zack but in which part of the question xml sample should I add this?