Loading Html file in WebView in xaml in UWP from app data local folder
To load the index.html
in WebView
while offline, you need to make sure all the resource used in index.html
are correctly located in app's LocalFolder. And all these content must be placed in a subfolder under the local folder.
Ref Remarks in WebView class:
To load uncompressed and unencrypted content from your app’s LocalFolder or TemporaryFolder data stores, use the Navigate method with a Uri that uses the ms-appdata scheme. The WebView support for this scheme requires you to place your content in a subfolder under the local or temporary folder. This enables navigation to URIs such as ms-appdata:///local/folder/file.html and ms-appdata:///temp/folder/file.html . (To load compressed or encrypted files, see NavigateToLocalStreamUri.)
For example, I created a simple index.html
that use index.js
in js
folder and index.css
in css
folder.
index.html
<!DOCTYPE html>
<html>
<head>
<title></title>
<meta charset="utf-8" />
<script src="js/index.js"></script>
<link href="css/index.css" rel="stylesheet" />
</head>
<body>
<button id="myBtn">Click Me!</button>
<div id="myDiv"></div>
</body>
</html>
index.js
window.onload = function () {
document.getElementById("myBtn").onclick = function () {
document.getElementById("myDiv").innerHTML += "You have clicked once! <br>";
}
}
index.css
#myDiv {
border: 2px dotted black;
width: 500px;
height: 500px;
}
They located in my app's LocalFolder like following:
Then in my UWP app, I used following code:
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<WebView x:Name="Browser" />
</Grid>
protected override void OnNavigatedTo(NavigationEventArgs e)
{
Browser.Navigate(new Uri("ms-appdata:///local/Downloads/index.html"));
}
This works well:
So if your html file is not loading, please check your app's LocalFolder and make sure your html file and the resources are located in right place.
On Local Machine, the data files are stored in the folder
%USERPROFILE%\AppData\Local\Packages\{PackageId}
which usually is C:\Users\{UserName}\AppData\Local\Packages\{PackageId}, where {UserName}
corresponds to the Windows user name and {PackageId}
corresponds to the Windows Store application package identifier which you can find as Package family name
in Packaging tab of your app's manifest file. The LocalState folder inside the package folder is the LocalFolder.
For Mobile Emulator, we can use some tools like IsoStoreSpy or Windows Phone Power Tools to check the LocalFolder.
If you can load the html file, but some resources are missing like missing the css style, you may need to check your html code and make sure the references are right.
Related videos on Youtube
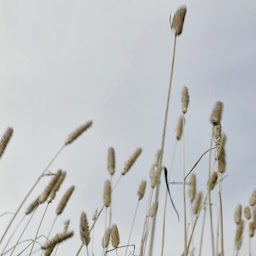
arun G
Experienced Software Development Engineer with a demonstrated history of working in different domains.Skilled in Angular,C#.
Updated on June 04, 2022Comments
-
arun G almost 2 years
I have a requirement where I need to load an html file from app data folder in xaml WebView in UWP. Html file is also referencing different Js files in another folder ("99/js/"). Any one with UWP knowledge guide me. Thanks in advance I am using following code,
Browser
is my WebView.var Uri = new Uri("ms-appdata:///Local/Downloads/99/index.html"); Browser.Navigate(Uri);
My folder structure in 99 folder is:
udapte
I am trying load html file in offline to WebView which is not loading same html file is loading with server url.-
Jay Zuo about 8 yearsPlease clarify your specific problem or provide additional details to highlight exactly what you need. As it's currently written, it's hard to tell exactly what you're asking.
-
-
testing over 7 yearsYou can also place the files at other locations. But this approach doesn't seem to work for xml with xsl files ...