Loading images for animation in UIImageView - iOS
Solution 1
You may try out the following code to load images into an array in a better way
- (void)viewDidLoad {
[super viewDidLoad];
NSMutableArray *imgListArray = [NSMutableArray array];
for (int i=1; i <= 300; i++) {
NSString *strImgeName = [NSString stringWithFormat:@"loading%03d.png", i];
UIImage *image = [UIImage imageNamed:strImgeName];
if (!image) {
NSLog(@"Could not load image named: %@", strImgeName);
}
else {
[imgListArray addObject:image];
}
}
imgView = [[UIImageView alloc] init];
[imgView setAnimationImages:imgListArray];
}
Solution 2
There is an easier way to do this. You can simply use:
[UIImage animatedImageNamed:@"loading" duration:1.0f]
Where 1.0f
is the duration to animate all the images. For this to work though, your images must be named like this:
loading1.png
loading2.png
.
.
loading99.png
.
.
loading300.png
That is, without padding with 0.
The function animatedImageNamed
is available from iOS 5.0 onwards.
Solution 3
Depending on the size of your images, a 300 image animation sequence may be quite the memory hog. Using a a movie might be a better solution.
Solution 4
Your code will crash when run on the device, it is just not possible to decompress that many images into memory on iOS. You will get memory warnings and then your app will be killed by the OS. See my answer to how-to-do-animations-using-images-efficiently-in-ios for a solution that will not crash on the device.
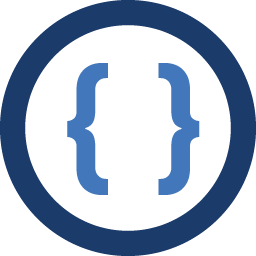
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I have around 300 images to be loaded for animation the images are named
loading001.png, loading002.png, loading003.png, loading004.png………loading300.png
I am doing it in the following way.
.h file
#import <UIKit/UIKit.h> @interface CenterViewController : UIViewController { UIImageView *imgView; } @end
.m file
@implementation CenterViewController - (void)viewDidLoad { [super viewDidLoad]; imgView = [[UIImageView alloc] init]; imgView.animationImages = [[NSArray alloc] initWithObjects: [UIImage imageNamed:@"loading001.png"], [UIImage imageNamed:@"loading002.png"], [UIImage imageNamed:@"loading003.png"], [UIImage imageNamed:@"loading004.png"], nil]; } - (IBAction)startAnimation:(id)sender{ [imgView startAnimating]; } @end
Is there a efficient way to load the images into a array. I had tried it with
for loop
but was not able to figure it out.