Loading Images into Div Dynamically
17,035
Solution 1
Try to use:
$("#divID").html("<img style='position:absolute' src='path/to/the/ajax_loader.gif'>");
If you using ajax:
function sendAjax()
{
$("#divID").html("<img style='position:absolute' src='path/to/the/ajax_loader.gif'>");
// you ajax code
$("#divID").html(ajaxResponse);
}
you can also use document.getElementById('divID').innerHTML
instead of $("#divID").html()
. its also work fine
If you use this method, doesn't need to hide the div using css. its automatically remove after the ajax response.
Solution 2
Well, you can try this:
<div id="desiredDiv">
</div>
<script>
var images = [
'http://www.example.com/img/image1.png',
'http://www.example.com/img/image2.png',
'http://www.example.com/img/image3.png',
...
];
for(var i=0; i<images.length; i++) {
$('#desiredDiv').append('<img src="'+images[i]+'" alt="" />');
}
</script>
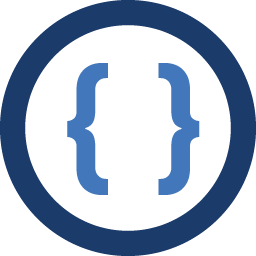
Author by
Admin
Updated on June 05, 2022Comments
-
Admin about 2 years
I have a list of profile images which appear in a "menu drop down" div which is initially hidden via CSS. I would like to load these images dynamically (as a list) when each menu item is selected, so as to reduce the page loading time. How is this possible?