Local and Push Notifications in IOS version compatible
Solution 1
IOS 12 :- Group notification
set threadIdentifier
UNMutableNotificationContent
to create group notification
create local notification group
let content = UNMutableNotificationContent()
content.title = "Group Notifications"
content.body = "Body of notification"
content.threadIdentifier = "group-identifire"
create remote notification group need to pass thread-id
in payload
{
"aps" : {
"alert" : {
"title" : "Group Notifications",
"body" : "Body of notification"
}
"thread-id" : "group-identifire"
}
}
IOS 11 :- You can also use following code for iOS 11. No any kind of changes requires in push and local notification
Creating A Notification Request
import UserNotifications
if #available(iOS 10.0, *) {
//iOS 10.0 and greater
UNUserNotificationCenter.current().delegate = self
UNUserNotificationCenter.current().requestAuthorization(options: [.badge, .sound, .alert], completionHandler: { granted, error in
DispatchQueue.main.async {
if granted {
UIApplication.shared.registerForRemoteNotifications()
}
else {
//Do stuff if unsuccessful...
}
}
})
}
else {
//iOS 9
let type: UIUserNotificationType = [UIUserNotificationType.badge, UIUserNotificationType.alert, UIUserNotificationType.sound]
let setting = UIUserNotificationSettings(types: type, categories: nil)
UIApplication.shared.registerUserNotificationSettings(setting)
UIApplication.shared.registerForRemoteNotifications()
}
Schedule Local Notification
if #available(iOS 10.0, *) {
//iOS 10 or above version
let center = UNUserNotificationCenter.current()
let content = UNMutableNotificationContent()
content.title = "Late wake up call"
content.body = "The early bird catches the worm, but the second mouse gets the cheese."
content.categoryIdentifier = "alarm"
content.userInfo = ["customData": "fizzbuzz"]
content.sound = UNNotificationSound.default()
var dateComponents = DateComponents()
dateComponents.hour = 15
dateComponents.minute = 49
let trigger = UNCalendarNotificationTrigger(dateMatching: dateComponents, repeats: true)
let request = UNNotificationRequest(identifier: UUID().uuidString, content: content, trigger: trigger)
center.add(request)
} else {
// ios 9
let notification = UILocalNotification()
notification.fireDate = NSDate(timeIntervalSinceNow: 5) as Date
notification.alertBody = "Hey you! Yeah you! Swipe to unlock!"
notification.alertAction = "be awesome!"
notification.soundName = UILocalNotificationDefaultSoundName
UIApplication.shared.scheduleLocalNotification(notification)
}
UIApplicationDelegate
func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
let tokenParts = deviceToken.map { data -> String in
return String(format: "%02.2hhx", data)
}
let token = tokenParts.joined()
print(token)
}
func application(_ application: UIApplication, didFailToRegisterForRemoteNotificationsWithError error: Error) {
}
UNUserNotificationCenterDelegate
Only available in ios 10 and above version
The method will be called on the delegate only if the application is in the foreground
You can present default banner with helping of following method
@available(iOS 10.0, *)
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
completionHandler([.badge,.alert,.sound])
}
The method will be called on the delegate when the user responded to the notification by opening the application, dismissing the notification or choosing a UNNotificationAction
@available(iOS 10.0, *)
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
}
Solution 2
I have made this class for Swift 3 which has a function for requesting permission to push notification and also a send notification. Works on for iOS 9 and iOS 10+.
import UIKit
import UserNotifications
class LocalNotification: NSObject, UNUserNotificationCenterDelegate {
class func registerForLocalNotification(on application:UIApplication) {
if (UIApplication.instancesRespond(to: #selector(UIApplication.registerUserNotificationSettings(_:)))) {
let notificationCategory:UIMutableUserNotificationCategory = UIMutableUserNotificationCategory()
notificationCategory.identifier = "NOTIFICATION_CATEGORY"
//registerting for the notification.
application.registerUserNotificationSettings(UIUserNotificationSettings(types:[.sound, .alert, .badge], categories: nil))
}
}
class func dispatchlocalNotification(with title: String, body: String, userInfo: [AnyHashable: Any]? = nil, at date:Date) {
if #available(iOS 10.0, *) {
let center = UNUserNotificationCenter.current()
let content = UNMutableNotificationContent()
content.title = title
content.body = body
content.categoryIdentifier = "Fechou"
if let info = userInfo {
content.userInfo = info
}
content.sound = UNNotificationSound.default()
let comp = Calendar.current.dateComponents([.hour, .minute], from: date)
let trigger = UNCalendarNotificationTrigger(dateMatching: comp, repeats: true)
let request = UNNotificationRequest(identifier: UUID().uuidString, content: content, trigger: trigger)
center.add(request)
} else {
let notification = UILocalNotification()
notification.fireDate = date
notification.alertTitle = title
notification.alertBody = body
if let info = userInfo {
notification.userInfo = info
}
notification.soundName = UILocalNotificationDefaultSoundName
UIApplication.shared.scheduleLocalNotification(notification)
}
print("WILL DISPATCH LOCAL NOTIFICATION AT ", date)
}
}
Usage:
You can request permission anywhere:
LocalNotification.registerForLocalNotification(on: UIApplication.shared)
And to dispatch a local notification:
LocalNotification.dispatchlocalNotification(with: "Notification Title for iOS10+", body: "This is the notification body, works on all versions", at: Date().addedBy(minutes: 2))
Tip:
You can set the notification to fire at any future Date, in this example I'm using a date extension to get a future date in minutes to the notification fire. This is it:
extension Date {
func addedBy(minutes:Int) -> Date {
return Calendar.current.date(byAdding: .minute, value: minutes, to: self)!
}
}
Related videos on Youtube
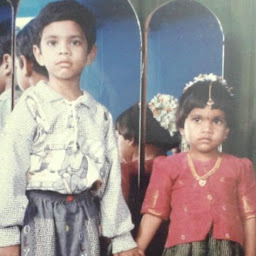
sindhu kopparati
Updated on July 09, 2022Comments
-
sindhu kopparati almost 2 years
I developed
local Notifications
iniOS 10
. It is working perfectly. But now how should i codelocal notifications
andpush notification
if user is usingiOS 9
and above versions. Can anyone help please?Below is code in
iOS 10
import UIKit import UserNotifications @available(iOS 10.0, *) class ViewController: UIViewController,UNUserNotificationCenterDelegate { override func viewDidLoad() { super.viewDidLoad() if #available(iOS 10.0, *) { //Seeking permission of the user to display app notifications UNUserNotificationCenter.current().requestAuthorization(options: [.alert,.sound,.badge], completionHandler: {didAllow,Error in }) UNUserNotificationCenter.current().delegate = self } } //To display notifications when app is running inforeground func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) { completionHandler([.alert, .sound, .badge]) } override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() // Dispose of any resources that can be recreated. } @IBAction func buttonPressed(_ sender: UIButton) { if #available(iOS 10.0, *) { //Setting content of the notification let content = UNMutableNotificationContent() content.title = "hello" content.body = "notification pooped out" content.badge = 1 //Setting time for notification trigger let date = Date(timeIntervalSinceNow: 10) var dateCompenents = Calendar.current.dateComponents([.year, .month, .day, .hour, .minute, .second], from: date) let trigger = UNCalendarNotificationTrigger(dateMatching: dateCompenents, repeats: false) //Adding Request let request = UNNotificationRequest(identifier: "timerdone", content: content, trigger: trigger) UNUserNotificationCenter.current().add(request, withCompletionHandler: nil) } } }
-
shallowThought about 7 yearsWhat is your issue? What have you tried?
-
sindhu kopparati about 7 yearsI need to modify my code such that it also works in iOS9. UserNotifications framework which i used here is only present in iOS 10
-
ArgaPK about 6 yearshow to set different date components or how to call local notfications multiple times?
-
-
ArgaPK about 6 yearshow to set different date components or how to call local notfications multiple times?