localhost didn't send any data - ERR_EMPTY_RESPONSE
36,126
Solution 1
Your courses
is an object. You need to send it as string over wire. Send it as json and the browser will be able to parse it.
app.get('/api/courses/', (req, res) => {
// change here, your object is stringified to json by express
res.json(courses);
});
To take input from browser, you'd have to use packages like body-parser
and not app.use(express.json)
var express = require('express')
var bodyParser = require('body-parser')
var app = express()
// parse application/x-www-form-urlencoded
app.use(bodyParser.urlencoded({ extended: false }))
// parse application/json
app.use(bodyParser.json())
Solution 2
I had a similar problem which was caused by calling an https only api endpoint using http.
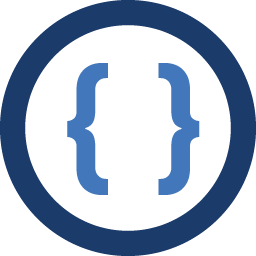
Author by
Admin
Updated on January 30, 2022Comments
-
Admin over 2 years
Using VScode to write NodeJS
But to check, localhost isn't responding properly, it says "the page didn't send any data. I tried looking everywhere and changed all the possible settings I can.
restored chrome settings cleared cache, cleared history, changed port numbers, changed LAN settings.
'''' const Joi = require('joi'); const express = require('express'); const app = express(); app.use(express.json); //to take inputs ffrom the browser const courses = [ {id: 1, name: "course 1"}, {id: 2, name: "course 2"}, {id: 3, name: "course 3"}, ]; app.get('/', (req, res) =>{ res.send("Hello world!!"); res.end(); }); app.get('/api/courses/', (req, res) =>{ res.send(courses); res.end(); }); const port = process.env.port || 8080; app.listen(port, ()=> console.log(`Listining on port ${port}..`)); ''''
Want to see all the courses printed on the webpage.
-
Admin about 5 yearsMan, it worked. Thank you so much, was working on it since morning. Another problem, I am using postman, but it's not working, do you have any idea? how to figure it out.
-
Admin about 5 yearsI figured out naga. Thank you.
-
Grant over 2 yearsHow did you get around it? I'm constantly getting an empty response, and it's not because of malformed data - I've brought a production app to development, I've set all of the development variables & the postgresql settings, the database is connecting, the only issue is "empty responses"
-
Thabiso Mofokeng over 2 yearsWhat is your design setup? Are you getting the empty response from an http api or standard web app?
-
Grant over 2 yearsThank you for your response @Thabiso - in the end it was because my Postgres credentials were incorrect. NestJS was only throwing an exception if the connection to the server was incorrect but not if the credentials didn't log into the table... weird but hey, problem solved due to
.env
variables/credentials & unrelated to https.