Login Form with Ajax using Laravel 5.2
Solution 1
As I understood Your code example is just copy of AuthenticatesUser
trait.
So to avoid big changes and make it work, just replace default controller code in app/Http/Controllers/LoginController.php
with this:
<?php
namespace App\Http\Controllers\Auth;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
class LoginController extends Controller
{
public function __construct()
{
$this->middleware('guest', ['except' => 'logout']);
}
protected function username() {
return 'email';
}
public function login(Request $request)
{
$credentials = $request->only($this->username(), 'password');
$authSuccess = Auth::attempt($credentials, $request->has('remember'));
if($authSuccess) {
$request->session()->regenerate();
return response(['success' => true], Response::HTTP_OK);
}
return
response([
'success' => false,
'message' => 'Auth failed (or some other message)'
], Response::HTTP_FORBIDDEN);
}
public function logout(Request $request)
{
Auth::logout();
$request->session()->flush();
$request->session()->regenerate();
return redirect('/');
}
}
js part can keep the same:
$.ajax({
type: "POST",
url: "/login",
data: formData,
dataType:'json',
success: function (response) {
if(response.success) {
location.reload();
}
},
error: function (jqXHR) {
var response = $.parseJSON(jqXHR.responseText);
if(response.message) {
alert(response.message);
}
}
});
but I personally prefer to handle not the button that does submit, but the form generally, to prevent this happen when user press enter
button than just click on the login button.
check this example:
html part:
<form class="login" action="{{ url('/login') }}" method="post" data-type="json">
<input type="text" name="email">
<input type="password" name="password">
<button type="submit">login</button>
</form>
js part:
$(function() {
$.ajaxSetup({
headers: {
'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content')
}
});
$('form.login:first').on('submit', function(e){
e.preventDefault();
var $this = $(this);
$.ajax({
type: $this.attr('method'),
url: $this.attr('action'),
data: $this.serializeArray(),
dataType: $this.data('type'),
success: function (response) {
if(response.success) {
location.reload();
}
},
error: function (jqXHR) {
var response = $.parseJSON(jqXHR.responseText);
if(response.message) {
alert(response.message);
}
}
});
});
});
Solution 2
You can try adding in jquery
dataType: 'JSON'
or Try to store in Session and use
Redirect::back()
or
return redirect($this->loginPath())
->withInput($request->only('email', 'remember'))
->withErrors([
'email' => $this->getFailedLoginMessage(),
]);
Solution 3
Please try this one
use Validator;
use Auth;
public function postUserLogin(Request $request) {
$credentials = array_trim($request->only('email', 'password'));
$rules = ['email' => 'required|email|max:255',
'password' => 'required'
];
$validation = Validator::make($credentials, $rules);
$errors = $validation->errors();
$errors = json_decode($errors);
if ($validation->passes()) {
if (Auth::attempt(['email' => trim($request->email),
'password' => $request->password,
], $request->has('remember'))) {
return response()->json(['redirect' => true, 'success' => true], 200);
} else {
$message = 'Invalid username or password';
return response()->json(['password' => $message], 422);
}
} else {
return response()->json($errors, 422);
}
}
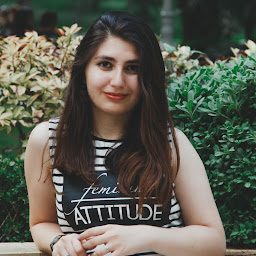
Nigar Jafar
Updated on June 17, 2022Comments
-
Nigar Jafar almost 2 years
I try to create login form with Ajax using Laravel 5.2 Auth.
$(document).ready(function(){ $.ajaxSetup({ headers: { 'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content') } }); $('#login').on('click',function(e){ e.preventDefault(); var formData = { email: $('#email').val(), password: $('#password').val(), } $.ajax({ type: "POST", url: "/login", data: formData, success: function (data) { location.reload(); }, error: function (data) { } }); }); })enter code here
Laravel default login function:
public function login(Request $request) { $this->validateLogin($request); // If the class is using the ThrottlesLogins trait, we can automatically throttle // the login attempts for this application. We'll key this by the username and // the IP address of the client making these requests into this application. $throttles = $this->isUsingThrottlesLoginsTrait(); if ($throttles && $lockedOut = $this->hasTooManyLoginAttempts($request)) { $this->fireLockoutEvent($request); return $this->sendLockoutResponse($request); } $credentials = $this->getCredentials($request); if (Auth::guard($this->getGuard())->attempt($credentials, $request->has('remember'))) { return $this->handleUserWasAuthenticated($request, $throttles); } // If the login attempt was unsuccessful we will increment the number of attempts // to login and redirect the user back to the login form. Of course, when this // user surpasses their maximum number of attempts they will get locked out. if ($throttles && ! $lockedOut) { $this->incrementLoginAttempts($request); } return $this->sendFailedLoginResponse($request); }
/login return index page as a response. I need json response about error messages or success message. It is said that changing Laravel core functions is not advisable. Then how can I get it?