Loop through an object and get the value from all its variables
You can use reflection to do this:
Field[] fields = games.getClass().getDeclaredFields();
for(Field field: fields) {
//do stuff
}
getDeclaredFields()
:
Returns an array of Field objects reflecting all the fields declared by the class or interface represented by this Class object. This includes public, protected, default (package) access, and private fields, but excludes inherited fields.
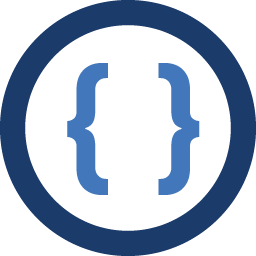
Admin
Updated on August 17, 2021Comments
-
Admin almost 3 years
I'm using Gson to parse an array of JSON objects to POJO. I am then using a
for-loop
to send each one of the parsed objects to a method for processing. I cannot seem to find a way to loop through the individual objects and get the values contained within.For instance:
private Double thing1; private Double thing2; private Dboule thing3; private void doSomething(MyObject myObj){ ...myObject contains thing1, thing2, thing3 which are each doubles. //I want to loop through the entire object, //grab thing1's value and do something with it, then grab thing2's value... etc. }
Is this easily done? I have been trying for a while now and I cannot seem to get it to work. I can grab them individually easily enough by using:
myObj.thing1
But it will need to be done in a loop as there are a TON of values coming in.
UPDATE: This ALMOST works but it prints the entire object and then it prints the individual object values. How can i get it to just print the individual values without first printing everything?
for(Field field : data.getClass().getDeclaredFields()){ Object value = field.get(data); System.out.println(value); }
Output:
[{"accel_1":0.012,"accel_2":0.125,"accel_3":0.03,"accel_4":0.012,"accel_5":0.125,"accel_6":0.03,"accel_7":0.012,"accel_8":0.125,"accel_9":0.03,"accel_10":0.012,"accel_11":0.125,"accel_12":0.03},{"accel_1":0.18,"accel_2":0.26,"accel_3":0.05,"accel_4":0.18,"accel_5":0.26,"accel_6":0.05,"accel_7":0.18,"accel_8":0.26,"accel_9":0.05,"accel_10":0.18,"accel_11":0.26,"accel_12":0.05},{"accel_1":0.06,"accel_2":0.02,"accel_3":0.03,"accel_4":0.06,"accel_5":0.02,"accel_6":0.03,"accel_7":0.06,"accel_8":0.02,"accel_9":0.03,"accel_10":0.06,"accel_11":0.02,"accel_12":0.03}] 0.012 0.125 0.03 0.012 0.125 0.03 0.012 0.125 0.03 0.012 0.125 0.03 [{"accel_1":0.012,"accel_2":0.125,"accel_3":0.03,"accel_4":0.012,"accel_5":0.125,"accel_6":0.03,"accel_7":0.012,"accel_8":0.125,"accel_9":0.03,"accel_10":0.012,"accel_11":0.125,"accel_12":0.03},{"accel_1":0.18,"accel_2":0.26,"accel_3":0.05,"accel_4":0.18,"accel_5":0.26,"accel_6":0.05,"accel_7":0.18,"accel_8":0.26,"accel_9":0.05,"accel_10":0.18,"accel_11":0.26,"accel_12":0.05},{"accel_1":0.06,"accel_2":0.02,"accel_3":0.03,"accel_4":0.06,"accel_5":0.02,"accel_6":0.03,"accel_7":0.06,"accel_8":0.02,"accel_9":0.03,"accel_10":0.06,"accel_11":0.02,"accel_12":0.03}] 0.18 0.26 0.05 0.18 0.26 0.05 0.18 0.26 0.05 0.18 0.26 0.05 [{"accel_1":0.012,"accel_2":0.125,"accel_3":0.03,"accel_4":0.012,"accel_5":0.125,"accel_6":0.03,"accel_7":0.012,"accel_8":0.125,"accel_9":0.03,"accel_10":0.012,"accel_11":0.125,"accel_12":0.03},{"accel_1":0.18,"accel_2":0.26,"accel_3":0.05,"accel_4":0.18,"accel_5":0.26,"accel_6":0.05,"accel_7":0.18,"accel_8":0.26,"accel_9":0.05,"accel_10":0.18,"accel_11":0.26,"accel_12":0.05},{"accel_1":0.06,"accel_2":0.02,"accel_3":0.03,"accel_4":0.06,"accel_5":0.02,"accel_6":0.03,"accel_7":0.06,"accel_8":0.02,"accel_9":0.03,"accel_10":0.06,"accel_11":0.02,"accel_12":0.03}] 0.06 0.02 0.03 0.06 0.02 0.03 0.06 0.02 0.03 0.06 0.02 0.03
Here are the Full Methods:
private void convertJSON(String jsonString) throws IllegalArgumentException, IllegalAccessException { Gson gson = new Gson(); Type data = new TypeToken<ArrayList<MyObject>>(){}.getType(); List<MyObject> fhd = gson.fromJson(jsonString, data); for(MyObject current : fhd){ printIt(current); } } public void printIt(MyObject data) throws IllegalArgumentException, IllegalAccessException{ for(Field field : data.getClass().getDeclaredFields()){ Object value = field.get(data); System.out.println(value); } }