loop through JPanel
Solution 1
for (Component c : pane.getComponents()) {
if (c instanceof JTextField) {
((JTextField)c).setText("");
}
}
But if you have JTextFields more deeply nested, you could use the following recursive form:
void clearTextFields(Container container) {
for (Component c : container.getComponents()) {
if (c instanceof JTextField) {
((JTextField)c).setText("");
} else
if (c instanceof Container) {
clearTextFields((Container)c);
}
}
}
Edit: A sample for Tom Hawtin - tackline suggestion would be to have list in your frame class:
List<JTextField> fieldsToClear = new LinkedList<JTextField>();
and when you initialize the individual text fields, add them to this list:
someField = new JTextField("Edit me");
{ fieldsToClear.add(someField); }
and when the user clicks on the clear button, just:
for (JTextField tf : fieldsToClear) {
tf.setText("");
}
Solution 2
Whilst another answer shows a direct way to solve your problem, your question is implying a poor solution.
Generally want static dependencies between layers to be one way. You should need to go a pack through getCommponents
. Casting (assuming generics) is an easy way to see that something has gone wrong.
So when you create the text fields for a form, add them to the list to be cleared in a clear operation as well as adding them to the panel. Of course in real code there probably other things you want to do to them too. In real code you probably want to be dealing with models (possibly Document
) rather than JComponent
s.
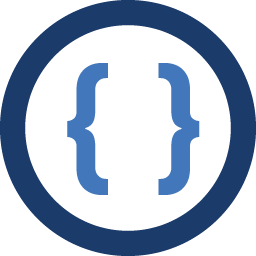
Admin
Updated on June 08, 2022Comments
-
Admin almost 2 years
In order to initialize all
JTextfField
s on aJPanel
when users click a "clear button", I need to loop through theJPanel
(instead of setting all individual field to "").How can I use a for-each loop in order to iterate through the
JPanel
in search ofJTextField
s? -
Brett almost 15 yearsDoes that clear combo boxes too? (Might as well be static, btw.)
-
akarnokd almost 15 yearsJComboBox (extends JComponent) and JTextField (extends JTextComponent which extends JComponent) are on two different paths
-
akarnokd almost 15 yearsI checked the source of JComboBox and I don't see any place where JComboBox adds its editor component to its components list.
-
Admin almost 15 yearsHi there, Thank a lot. This sounds impressive but I would not know how to implement your solution. Comcrete examples are worth a 1000 words. Very much appreciated. Dallag.
-
Admin almost 15 yearsThank you so very much kd304 it worked a treat. Just had to add import java.awt.*; import javax.swing.*; import java.awt.Component;
-
akarnokd almost 15 yearsYes, sorry. I'm too customed to CTRL+SHIFT+O in Eclipse to organize my imports.
-
akarnokd almost 15 yearsAnd to push it further, I once tried an annotation based solution for fun. I annotated my fields in the class with my @SaveContent and used a reflective approach to load/save contents of the annotated components.
-
BNetz about 5 yearsThanks for the hint how to loop recursively through nested Components