Looping through a datatable and remove row
Solution 1
If dr.Length is zero, then your select did not return any rows. If you want to delete rows that don't match your criteria, then I would implement that as a different type of query. It could also be that I am completely misunderstanding your question. Could you update your question to show your SQL and indicate where in the code sample you are having the problem: inside the loop or after the loop where you have your comment?
Solution 2
I'm not sure I completely understand your question but it seems that you try to remove an entry from the collection while you are still looping over it. (which will cause an array index error)
You should save a reference to each entry you want to delete in a new collection and then remove all the new entries from the old collection:
DataRow[] dr = payments.dtPayments.Select(myselect);
List<DataRow> rowsToRemove = new List<DataRow>();
for (int a = 0; a < dr.Length; a++) {
if(/* You want to delete this row */) {
rowsToRemove.Add(dr[a]);
}
}
foreach(var dr in rowsToRemove) {
payments.dtPayments.Rows.Remove(dr);
}
Solution 3
You need to create a datarow outside the loop, then when you get to the row you want to remove assign it to the row you created outside. and break the loop. Then below the loop you can remove it.
DataRow r = null;
foreach(DataRow row in table.Rows)
{
if(condition==true)
{
r = row;
break;
}
}
table.Rows.Remove(r);
Solution 4
You can loop through the collection in reverse (for (int i=rows.length-1;x>-1;x--) ) to remove multiple rows to ensure you won't get index out of bound errors.
Solution 5
Have you tryed this?
payments.dtPayments.Rows.Remove(dr)
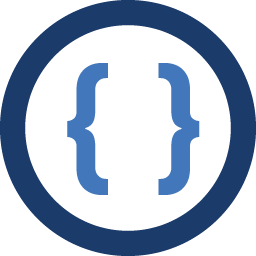
Admin
Updated on November 04, 2020Comments
-
Admin over 3 years
I have populated a Datatable, from 2 different servers. I am able to make adjustments where my length>0, what I want to do is remove the rows that does not hit. Here is a summary of what I have
DataRow[] dr = payments.dtPayments.Select(myselect); if (dr.Length > 0) { for (int a = 0; a < dr.Length; a++) { if (thisOption == true) dr[0].Delete(); else if (otherOption == true) { dr[0]["Date"] = myDataReader["date"].ToString().Trim(); dr[0]["Pay"] = payTypeName(myDataReader["ccdsrc"].ToString() .Trim()); } } } if (dr.Length == 0) { if (LastOption == true) { //DataRow should be removed } }
-
Admin over 15 yearsI did, and I looked more into it. I first had dr[0].delete, I had what you suggested(both dr and dr[0]) and the error index was outside the bounds of the array is my error. Is there a way to correct that?
-
Admin over 15 yearsyou are right. I realize that I am not returning any rows. What I did was make a new dataset and populate the rows with what I DID find. Thanks
-
Richard over 11 yearsI like this a lot better than the double loop. This is always my preferred method.