<Python> Two iterating variables in a for loop
In your code, you use the enumerate()
function. You can imagine that it does the following:
>>> print enumerate("a","b","c")
>>> [(0,"a"),(1,"b"),(2,"c")]
(This isn't exactly correct, but it is close enough.)
enumerate()
turns a list of elements into a list* of tuples, where each tuple consists of the index of the element and the element. Tuples are similar to lists.
*In fact it returns an iterator, not a list, but that shouldn't bother you too much.
But how do the two iteration variables work?
Let me illustrate this with a few examples.
In the first example, we iterate over each element in a list of integers.
simple_list = [1,2,3,4]
for x in simple_list:
print x
But we can also iterate over each element in a list of tuples of integers:
tuple_list = [(1,5),(2,6),(3,7),(4,8)]
for x, y in tuple_list:
print x, y
print x+y
Here, each element of the list is a tuple of two integers. When we write for tup in tuple_list
, then tup
is populated on each iteration with one tuple of integers from the list.
Now, because Python is awsome, we can "catch" the tuple of two integers into two different integer variables by writing (x,y)
(or x,y
, which is the same) in place of of tup
Does this make sense?
Analogously, we can iterate over 3-tuples:
tuple_list = [(1,5,10),(2,6,11),(3,7,12),(4,8,69)]
for x, y, z in tuple_list:
print x, y, z
print x+y-z
All of this works. Or should work (haven't run the code).
And of course,
tuple_list = [(1,5),(2,6),(3,7),(4,8)]
for tuple in tuple_list:
print tuple
also works.
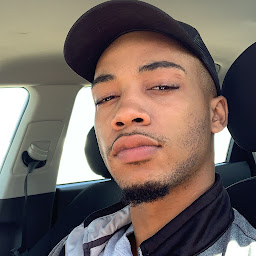
Jarrall Barnett
Updated on August 01, 2022Comments
-
Jarrall Barnett almost 2 years
Let me start of with the generic I'm a beginner with Python introduction. Hi, I'm a beginner with Python so please keep responses as closely aligned with plain English as possible :)
I keep running into these for loops where there are two iterating variables in a for loop. This is highly confusing to me because I just got my head wrapped around the basic concept of for loops. That is your iterating variable runs through one piece at a time through the for loop line by line (in most cases). So what then does two iterating variables do? I have some speculations but I'd like correct answers to put my thinking in the right direction.
Would someone type how the for loops would be read (in speaking terms) and explain what exactly is happening.
>>> elements = ('foo', 'bar', 'baz') >>> for elem in elements: ... print elem ... foo bar baz >>> for count, elem in enumerate(elements): ... print count, elem ... 0 foo 1 bar 2 baz
-
Jarrall Barnett over 8 yearsThat really made sense for me! I would rate up but I'm not at 15 reputation points yet.
-
Akmal Salikhov over 5 yearshow about this syntax:
arr = [[1, 2],[3, 4], [5]]; for i, v in arr: print (i, v)
? It looks like it flattens array of arrays...