maintain session between the pages in php?
Solution 1
There are two ways with which you can do this
- Sessions
- Hidden input fields
Sessions
To pass data from one page to another, you first need to call session_start()
on all pages that are going to use $_SESSION
superglobal variable.
Then you can store your values in sessions by using
$_SESSION['name'] = $_POST['name'];
$_SESSION['address'] = $_POST['address'];
$_SESSION['pin'] = $_POST['pin'];
To use these values in the second page, simply call them by their names. Ex:
$name = $_SESSION['name']; // will contain the value entered in first page
==================================================================================
Hidden Input Fields
This is more of a tedious approach but it does the job none the less. The process involves storing data that needs to be passed onto to different pages in hidden fields and later accessing them via the $_POST
or $_GET
superglobal.
page1.php (which posts to page2.php)
<input type="text" value="Page 1 content" name="content" />
<input type="text" value="Page 1 body" name="body" />
page2.php (which posts to page3.php)
<input type="hidden" value="<?php echo $_POST['content']; ?>" name="content" />
<input type="hidden" value="<?php echo $_POST['body']; ?>" name="body" />
<input type="text" value="Page 2 content" name="content2" />
<input type="text" value="Page 2 body" name="body2" />
page3.php
echo $_POST['content']; // prints "Page 1 content"
echo $_POST['body']; // prints "Page 1 body"
echo $_POST['content2']; // prints "Page 2 content"
echo $_POST['body2']; // prints "Page 2 body"
Solution 2
just use the below code in your first page <?php session_start(); ?>
and use the following code in your sencond page
<?php
$name = $_SESSION['name'];
$address = $_SESSION['address'];
$pin = $_SESSION['pin'];
echo $name."<br/>";
echo $address."<br/>";
echo $pin."<br/>";
?>
or you can use post
or get
method as below
For GET Method
<?php
$name = $_GET['name'];
$address = $_GET['address'];
$pin = $_GET['pin'];
echo $name."<br/>";
echo $address."<br/>";
echo $pin."<br/>";
?>
For POST METHOD
<?php
$name = $_POST['name'];
$address = $_POST['address'];
$pin = $_POST['pin'];
echo $name."<br/>";
echo $address."<br/>";
echo $pin."<br/>";
?>
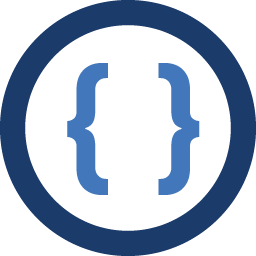
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am very new to PHP.
I want to transfer data between pages.
In my requirements I have the home page first, in that I have 3 fields: name, address, pin and then submit button.
When I entered the above fields and then click on submit, it moves to
page2.php
, it has form data.I have transferred the first form data to second page. Now in second page I have a submit button. When I click on that button the data is submitted to a MySQL database.
My problem is, how can I move first page values to
insertdata.php
page and submit the data ?