Make a label update while dragging a slider
33,382
Solution 1
You just need to change the valueChangingProperty()
to valueProperty()
and TADA, it works as you want !
A small sample is attached here :
import javafx.application.Application;
import javafx.beans.value.ChangeListener;
import javafx.beans.value.ObservableValue;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.Slider;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Demo extends Application {
@Override
public void start(Stage primaryStage) {
// Add a scene
VBox root = new VBox();
Scene scene = new Scene(root, 500, 200);
final Label betLabel = new Label("sdsd");
final Slider betSlider = new Slider();
betSlider.valueProperty().addListener(new ChangeListener<Number>() {
@Override
public void changed(
ObservableValue<? extends Number> observableValue,
Number oldValue,
Number newValue) {
betLabel.textProperty().setValue(
String.valueOf(newValue.intValue());
}
}
});
root.getChildren().addAll(betSlider, betLabel);
betLabel.textProperty().setValue("abc");
// show the stage
primaryStage.setTitle("Demo");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Solution 2
Bind the label's textProperty to the slider's valueProperty.
A format conversion is required in the binding to make it work.
Either Itachi's valueProperty() ChangeListener or a binding will work.
import javafx.application.Application;
import javafx.beans.binding.Bindings;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Slide extends Application {
@Override public void start(Stage stage) {
Label label = new Label();
Slider slider = new Slider(1, 11, 5);
label.textProperty().bind(
Bindings.format(
"%.2f",
slider.valueProperty()
)
);
VBox layout = new VBox(10, label, slider);
layout.setStyle("-fx-padding: 10px; -fx-alignment: baseline-right");
stage.setScene(new Scene(layout));
stage.setTitle("Goes to");
stage.show();
}
public static void main(String[] args) { launch(args); }
}
Solution 3
And if you want to do completely in in FXML, you can do this:
<TextField prefWidth="50" text="${speedSlider.value}"/>
<Slider fx:id="speedSlider" orientation="HORIZONTAL" prefWidth="300"
min="60" max="100000" blockIncrement="100"/>
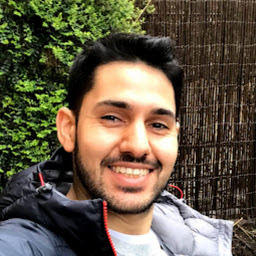
Author by
David Tzoor
Updated on February 04, 2021Comments
-
David Tzoor over 3 years
I'm using a
Slider
in my javaFX project and I have aLabel
that updates when I move the slider.I want the
Label
to update whilst I'm dragging theSlider
and not only when the drag is dropped.This is my code:
betSlider.valueChangingProperty().addListener(new ChangeListener<Boolean>() { @Override public void changed(ObservableValue<? extends Boolean> source, Boolean oldValue, Boolean newValue) { betLabel.textProperty().setValue(String.valueOf((int)betSlider.getValue())); } });
-
Jonathan Woollett-light about 5 yearsUsing this, the
TextField
can no longer be changed, can you give a version where changing theTextField
will also change theSlider
? -
Jeremy Caney over 3 yearsWelcome, and thank you for contributing an answer. Would you kindly edit your answer to to include an explanation of your code? That will help future readers better understand what is going on, and especially those members of the community who are new to the language and struggling to understand the concepts. That's especially true here, since your answer is competing for attention with five well-established answers.