Make Directory in python
16,892
You need to use expanduser to expand the '~' path
Here's the code you need
import os
from os.path import expanduser
home = expanduser('~')
dl_path = home + '/Downloads/PDMB'
def main():
if not os.path.exists(dl_path):
print "path doesn't exist. trying to make"
os.makedirs(dl_path)
if __name__ == '__main__':
main()
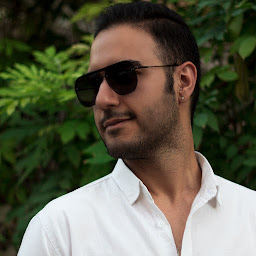
Author by
Ali Salehi
Updated on June 26, 2022Comments
-
Ali Salehi almost 2 years
I want to make a directory in Python.
Here is my code:
dl_path = "~/Downloads/PDMB" def main(): if not os.path.exists(dl_path): print "path doesn't exist. trying to make" os.makedirs(dl_path) if __name__ == '__main__': main()
I want that pdmb be in Download folder in
$HOME
(by the way my OS is Ubuntu), but it makes Home/Downloads/pdmb in the same folder that my code is.what should I do?
-
Matthias Fripp over 6 yearsIt would be simpler just to replace
dl_path = "~/Downloads/PDMB"
in the original code withdl_path = os.path.expanduser("~/Downloads/PDMB")
(or better yet,dl_path = os.path.join(os.path.expanduser("~"), "Downloads", "PDMB")
), since @ali-salehi is already accessingos.path
.