Make GDB print control flow of functions as they are called
Solution 1
In your case I would turn to the define
command in gdb, which allows you to define a function, which can take up to 10 arguments.
You can pass in the names of functions to "trace" as arguments to the function you define, or record them all in the function itself. I'd do something like the following
define functiontrace
if $arg0
break $arg0
commands
where
continue
end
end
if $arg1
...
Arguments to a user-defined function in gdb are referenced as $arg0-$arg9. Alternatively, you could just record every function you wanted to trace in the function, instead of using $arg0-9.
Note: this will not indent as to depth in the stack trace, but will print the stack trace every time the function is called. I find this approach more useful than strace etc... because it will log any function you want, system, library, local, or otherwise.
Solution 2
There's rbreak
cmd accepting regular expression for setting breakpoints. You can use:
(gdb) rbreak Foo.*
(gdb) rbreak Bar.*
(gdb) break printf
See this for details on breakpoints.
Then use commands
to print every function as it's called. E.g. let α = the number of the last breakpoint (you can check it with i br
if you missed), then do:
(gdb) commands 1-α
Type commands for breakpoint(s) 1-α, one per line.
End with a line saying just "end".
>silent
>bt 1
>c
>end
(gdb)
Some elaboration: silent
suppresses unnecessary informational messages, bt 1
prints the last frame of backtrace (i.e. it's the current function), c
is a shortcut for continue
, to continue execution, and end
is just the delimiter of command list.
NB: if you trace library functions, you may want to wait for lib to get loaded. E.g. set a break to main
or whatever function, run app until that point, and only then set breakpoints you wanted.
Solution 3
Use the right tool for the job ;)
How to print the next N executed lines automatically in GDB?
Solution 4
Did you see litb's excellent anwser to a similar post here ?
He uses readelf to get interesting symbols, gdb commands to get the trace, and awk to glue all that.
Basically what you have to change is to modify his gdb command script to remove the 1 depth from backtrace to see the stack and filter specific functions, and reformat the output with an awk/python/(...) script to present it as a tree. (I admit I'm too lazy to do it now...)
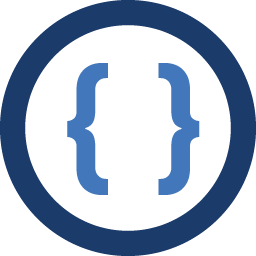
Admin
Updated on April 24, 2020Comments
-
Admin about 4 years
How do I make gdb print functions of interest as they are called, indented according to how deep in the stack they are?
I want to be able to say something like (made up):
(gdb) trace Foo* Bar* printf
And have gdb print all functions which begin with Foo or Bar, as they are called. Kind of like gnu cflow, except using the debugging symbols and only printing functions which actually get called, not all possible call flows.
Tools which won't help include cachegrind, callgrind and oprofile, which order the results by which functions were called most often. I need the order of calling preserved.
The wildcarding (or equivalent) is essential, as there are a lot of Foo and Bar funcs. Although I would settle for recording absolutely every function. Or, perhaps telling gdb to record all functions in a particular library.
Some GDB wizard must have a script for this common job!