Make Strings In List Uppercase - Python 3
Solution 1
Since the upper()
method is defined for string only and not for list, you should iterate over the list and uppercase each string in the list like this:
def to_upper(oldList):
newList = []
for element in oldList:
newList.append(element.upper())
return newList
This will solve the issue with your code, however there are shorter/more compact version if you want to capitalize an array of string.
map function
map(f, iterable)
. In this case your code will look like this:words = ['stone', 'cloud', 'dream', 'sky'] words2 = list(map(str.upper, words)) print (words2)
List comprehension
[func(i) for i in iterable]
.In this case your code will look like this:words = ['stone', 'cloud', 'dream', 'sky'] words2 = [w.upper() for w in words] print (words2)
Solution 2
You can use the list comprehension notation and apply theupper
method to each string in words
:
words = ['stone', 'cloud', 'dream', 'sky']
words2 = [w.upper() for w in words]
Or alternatively use map
to apply the function:
words2 = list(map(str.upper, words))
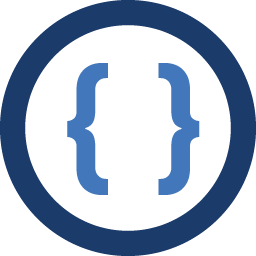
Admin
Updated on June 07, 2022Comments
-
Admin almost 2 years
I'm in the process of learning python and with a practical example I've come across a problem I cant seem to find the solution for. The error I get with the following code is
'list' object has to attribute 'upper'
.def to_upper(oldList): newList = [] newList.append(oldList.upper()) words = ['stone', 'cloud', 'dream', 'sky'] words2 = (to_upper(words)) print (words2)