MALICIOUS_CODE EI_EXPOSE_REP Medium
Solution 1
I think the key here is the if:
If instances are accessed by untrusted code, and unchecked changes to the mutable object would compromise security or other important properties, you will need to do something different.
So in other words, if you wanted an immutable object (i.e. you didn't have a setBirthdate()
method), your code be incorrect, because someone could write:
Date date = user.getBirthDate();
date.setMonth(1); // mutated!
So you would probably want the following instead:
public Date getBirthDate()
{return new Date(birthDate.getTime());} // essentially a clone
Solution 2
Yeah, I wouldn't really call it a ‘security’ issue as such... I mean, what attacker exactly is going to be writing malicious code against your objects? The real problem would be that you're quite likely yourself to trip up by accidentally calling getBirthDate
then modifying the result.
For this reason, it is common to have your getter clone mutable objects like Date
for returning, when you're using them as value types.
(You could also argue that Java's Date
shouldn't have been made mutable, but there's not much can be done about that now.)
Solution 3
Adding to the good answer of Matt Solnit, I've faced the same problem when setting a attribute, so I did the same:
public void setDataEmissaoNota (Date dataEmissaoNota)
{
this.dataEmissaoNota = new Date(dataEmissaoNota.getTime());
}
Work's fine!
Solution 4
Well, I'd say that all depends. There are other non security-related reasons to return immutable objects, since it may also lead to some hard-to-find bugs in your code if the object is misused.
Is the class going to be accessed by untrusted code and/or data? If so, you need to have a clear idea of where the responsibility lies in your application with regards to validating input.
Also, what is the nature of the application? If it's e.g. an externally accessible network service then the input should almost certainly be considered potentially malicious. However if it's an application run locally with no priviliges which gets input from a trusted source, then probably no need to worry.
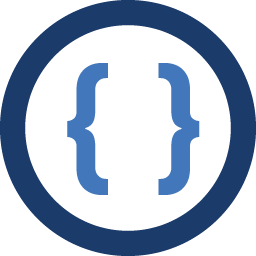
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I run findbugs against all of my code and only tackle the top stuff. I finally got the top stuff resolved and now am looking at the details. I have a simple entity, say a user:
public class User implements Serializable { protected Date birthDate; public Date getBirthDate() {return(birthDate);} public void setBirthDate(final Date birthDate) {this.birthDate = birthDate;} }
This class is incomplete, so don't harp me about it missing the
serialVersionUID
and other standard stuff, I am just concerned with thebirthDate
security hole.Now, according to the findbugs report, since I am returning a reference to a mutable object, that is a potential security risk. In practice though, how much does that really matter?
http://findbugs.sourceforge.net/bugDescriptions.html#EI_EXPOSE_REP
I suppose I still don't really see what the problem is here in this case. Should I pass in a
long
and set the date from that?Walter