Map.Entry: How to use it?
Solution 1
Map.Entry
is a key and its value combined into one class. This allows you to iterate over Map.entrySet()
instead of having to iterate over Map.keySet()
, then getting the value for each key. A better way to write what you have is:
for (Map.Entry<String, JButton> entry : listbouton.entrySet())
{
String key = entry.getKey();
JButton value = entry.getValue();
this.add(value);
}
If this wasn't clear let me know and I'll amend my answer.
Solution 2
Note that you can also create your own structures using a Map.Entry as the main type, using its basic implementation AbstractMap.SimpleEntry. For instance, if you wanted to have an ordered list of entries, you could write:
List<Map.Entry<String, Integer>> entries = new ArrayList<>();
entries.add(new AbstractMap.SimpleEntry<String, Integer>(myStringValue, myIntValue));
And so on. From there, you have a list of tuples. Very useful when you want ordered tuples and a basic Map is a no-go.
Solution 3
This code is better rewritten as:
for( Map.Entry me : entrys.entrySet() )
{
this.add( (Component) me.getValue() );
}
and it is equivalent to:
for( Component comp : entrys.getValues() )
{
this.add( comp );
}
When you enumerate the entries of a map, the iteration yields a series of objects which implement the Map.Entry
interface. Each one of these objects contains a key and a value.
It is supposed to be slightly more efficient to enumerate the entries of a map than to enumerate its values, but this factoid presumes that your Map
is a HashMap
, and also presumes knowledge of the inner workings (implementation details) of the HashMap
class. What can be said with a bit more certainty is that no matter how your map is implemented, (whether it is a HashMap
or something else,) if you need both the key and the value of the map, then enumerating the entries is going to be more efficient than enumerating the keys and then for each key invoking the map again in order to look up the corresponding value.
Solution 4
A Map is a collection of Key + Value pairs, which is visualized like this:
{[fooKey=fooValue],barKey=barValue],[quxKey=quxValue]}
The Map interface allows a few options for accessing this collection: The Key set [fooKey, barKey,quxKey]
, the Value set [fooValue, barValue, quxValue]
and finally entry Set [fooKey=fooValue],barKey=barValue],[quxKey=quxValue]
.
Entry set is simply a convenience to iterate over the key value pairs in the map, the Map.Entry is the representation of each key value pair. An equivalent way to do your last loop would be:
for (String buttonKey: listbouton.keySet()) {
this.add(listbouton.get(buttonKey)) ;
}
or
for (JButton button: listbouton.values()) {
this.add(button) ;
}
Solution 5
A Map consists of key/value pairs. For example, in your code, one key is "Add" and the associated value is JButton("+"). A Map.Entry is a single key/value pair contained in the Map. It's two most-used methods are getKey() and getValue()
. Your code gets all the pairs into a Set:
Set entrys = listbouton.entrySet() ;
and iterates over them. Now, it only looks at the value part using me.getValue()
and adds them to your PanneauCalcul
this.add((Component) me.getValue()) ; //don't understand
Often this type of loop (over the Map.Entry) makes sense if you need to look at both the key and the value. However, in your case, you aren't using the keys, so a far simpler version would be to just get all the values in your map and add them. e.g.
for (JButton jb:listbouton.values()) {
this.add(jb);
}
One final comment. The order of iteration in a HashMap is pretty random. So the buttons will be added to your PanneauCalcul in a semi-random order. If you want to preserve the order of the buttons, you should use a LinkedHashMap.
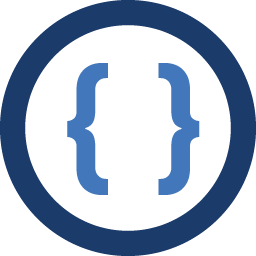
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'm working on creating a calculator. I put my buttons in a
HashMap
collection and when I want to add them to my class, which extendsJPanel
, I don't know how can I get the buttons from my collection. So I found on the internet the 2 last lines of my code, but I don't know their meaning.Here is my code:
import java.awt.Component; import java.util.HashMap; import java.util.Iterator; import java.util.Map; import java.util.Set; import javax.swing.JButton; import javax.swing.JPanel; public class PanneauCalcul extends JPanel { private HashMap<String, JButton> listbouton = new HashMap<String, JButton>() ; public PanneauCalcul() { for(int i = 0; i < 10; i ++) { listbouton.put("num" + i, new JButton("" + i)) ; } listbouton.put("add", new JButton("+")) ; listbouton.put("soustract", new JButton("-")) ; listbouton.put("multiply", new JButton("x")) ; listbouton.put("divise", new JButton("/")) ; listbouton.put("equal", new JButton("=")) ; Set entrys = listbouton.entrySet() ; Iterator iter = entrys.iterator() ; while(iter.hasNext()) { Map.Entry me = (Map.Entry)iter.next(); //don't understand this.add((Component) me.getValue()) ; //don't understand } EcouteCalcul ecout = new EcouteCalcul(this) ; } }
I don't understand how can we use
Map.Entry
--which is an interface--without redefiningMap.Entry
's functions.