Match and update values using linq from two lists?
Use Enumerable.Join to get items which match in both lists:
from x in firstlist
join y in secondList
on x.code equals y.Code
select new {
x.Name,
code = String.Format("{0} {1}", y.Code, y.description)
}
Updating objects in first list:
var query = from x in firstlist
join y in secondList
on x.code equals y.Code
select new { x, y };
foreach(var match in query)
match.x.Code = String.Format("{0} {1}", match.y.Code, match.y.description);
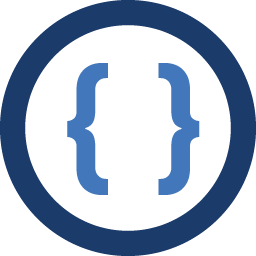
Admin
Updated on January 18, 2020Comments
-
Admin over 4 years
Hi I am new to the c# and
Linq
. I am trying to update the one list if the value of some property matches the value of the other lists property.Suppose i have one list as
firstlist
Name code ABC 101 DEF 201 GHI 301 JKL 401 MNO 101
And Second list as
secondlist
description Code IT 101 ADMIN 201 Develeopment 301 Testing 401 Service 501
And i want my resultant list as
Name code ABC 101 IT DEF 201 ADMIN GHI 301 Develeopment JKL 401 Testing MNO 101 IT
I tried something like this ,
var query = firstlist.Select(x => { x.Code = 101; return x.Code.ToString()+"IT"; })
But i want to match the code from the
secondlist
in place of101
and for matching code i want to update thefirstlist
code with thecode+description
i don't have any idea how to do it if anyone suggest any way or link which will guide me would be great.*******UPDATE*******
What i want to say is that as @Sergey suggested
from x in firstlist join y in secondList on x.code equals y.Code select new { x.Name, code = String.Format("{0} {1}", y.Code, y.description) }
in place of this can i just do something like this
from x in firstlist join y in secondList on x.code equals y.Code select x.code = String.Format("{0} {1}", y.Code, y.description)
which will only update the existing
listone
in place of the creating new entity for each match -
Admin over 10 yearswhat if i have the very long list of properties in the
listone
do i have to mention all of them in thiscode = String.Format("{0} {1}", y.Code, y.description),someprop1,someprop2,someprop3
like this -
Sergey Berezovskiy over 10 years@Curiosity if you are talking about
firstlist
and properties ofx
then you can simply selectx
entity in result:select new { x, y }
-
Sergey Berezovskiy over 10 years@Curiosity where someprop1, someprop2 comes from?
-
Admin over 10 yearsThey are extra properties in the
firstlist
other than name and code so what i am saying is in place creating new entity for each match can i just update the existing value of thecode
by doing something likex.code=y.code+y.description
-
Sergey Berezovskiy over 10 years@Curiosity if you want to update items from firstlist, then you can enumerated results of query and update x.Code