Matlab - solving a third order differential equation
10,878
To use ODE45 (or similar) you need to convert the third order ODE into a system of first order ODEs.
To do so, let
y0 = y
y1 = y0'
y2 = y1'
y3 = y2'
Then
y0' = y1
y1' = y2
y2' = y3
and
y3' = y''' = -41*y2-360y1-900y0+600dx+1200x
you can now use ODE45 to integrate the system by nesting the function where x(t) and dx(t) are available.
function test()
% some random x function
x = @(t) exp(-t);
dx = @(t) -exp(-t);
% integrate numerically
[T, Y] = ode45(@linearized, [0 1], [2 1 -0.05 0]);
% plot the result
plot(T, Y(:,1))
% linearized ode
function dy = linearized(t,y)
dy = zeros(4,1);
dy(1) = y(2);
dy(2) = y(3);
dy(3) = y(4);
dy(4) = -41*y(3)-360*y(2)-900*y(1)+600*dx(t)+1200*x(t);
end
end
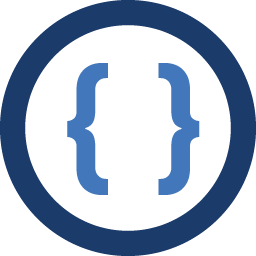
Author by
Admin
Updated on June 08, 2022Comments
-
Admin almost 2 years
y''' + 41y'' + 360y' + 900y = 600x' + 1200x; y(0)= 2 ; y'(0)= 1 ; y''(0) = -0.05
How can I solve this equation using the ODE45 function?
I tried this:
==> function dydt=f(t,y) dydt = [y(2) ; y(3) ; -41*y(3)-360*y(2)- 900*y(1)] ==> clear all; timerange=[0 1.4]; %seconds initialvalues=[2 1 -0.05]; [t,y]=ode45(@dydt, timerange, initialvalues) plot(t,y(:,1));
But I need put the X part in the equation - I don't know how...