Matrix Mirroring in python
Solution 1
This can be accomplished using a simple helper function in pure python:
def mirror(seq):
output = list(seq[::-1])
output.extend(seq[1:])
return output
inputs = [
['a', 'b', 'c'],
['d', 'e', 'f'],
['g', 'h', 'i'],
]
print(mirror([mirror(sublist) for sublist in inputs]))
Obviously, once the mirrored list is created, you can use it to create a numpy array or whatever...
Solution 2
Use numpy.lib.pad
with 'reflect'
m = [['a', 'b', 'c'],
['d', 'e', 'f'],
['g', 'h', 'i']]
n=np.lib.pad(m,((2,0),(2,0)),'reflect')
n
Out[8]:
array([['i', 'h', 'g', 'h', 'i'],
['f', 'e', 'd', 'e', 'f'],
['c', 'b', 'a', 'b', 'c'],
['f', 'e', 'd', 'e', 'f'],
['i', 'h', 'g', 'h', 'i']],
dtype='<U1')
Solution 3
import numpy as np
X= [[1, 2, 3],
[4, 5, 6],
[7, 8, 9]]
A = np.asanyarray(X)
B= np.flipud(A)
C= np.concatenate((B, A[1:]), axis=0)
D = C[:,1:]
F = np.fliplr(C)
E = np.concatenate((F, D), axis=1)
print(E)
I have added step by step transformation. flipud and flipud refrence
output
[[9 8 7 8 9]
[6 5 4 5 6]
[3 2 1 2 3]
[6 5 4 5 6]
[9 8 7 8 9]]
Solution 4
And here is a non-numpy solution:
a = [['a', 'b', 'c'], ['d', 'e', 'f'], ['g', 'h', 'i']]
b = list(reversed(a[1:])) + a # vertical mirror
c = list(zip(*b)) # transpose
d = list(reversed(c[1:])) + c # another vertical mirror
e = list(zip(*d)) # transpose again
Solution 5
Suppose you have
from numpy import array, concatenate
m = array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
You can invert this along the first (vertical) axis via
>>> m[::-1, ...]
array([[7, 8, 9],
[4, 5, 6],
[1, 2, 3]])
where ::-1
selects the rows from last to first in steps of -1
.
To omit the last row, explicitly ask for the selection to stop immediately before 0
:
>>> m[:0:-1, ...]
array([[7, 8, 9],
[4, 5, 6]])
This can then be concatenated along the first axis
p = concatenate([m[:0:-1, ...], m], axis=0)
to form:
>>> p
array([[7, 8, 9],
[4, 5, 6],
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
This can be repeated along the other axis too:
q = concatenate([p[..., :0:-1], p], axis=1)
yielding
>>> q
array([[9, 8, 7, 8, 9],
[6, 5, 4, 5, 6],
[3, 2, 1, 2, 3],
[6, 5, 4, 5, 6],
[9, 8, 7, 8, 9]])
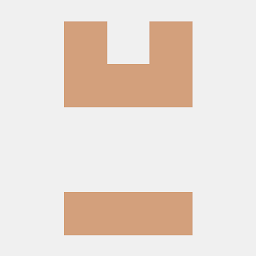
Sterling Butters
I am a recent college graduate from Texas A&M university with a BS in Nuclear Engineering. I find programming and development an intriguing hobby that can be largely applicable to my profession. I enjoy Python the most however I impressed more with the functionalities of Javascript.
Updated on June 27, 2022Comments
-
Sterling Butters almost 2 years
I have a matrix of numbers:
[[a, b, c] [d, e, f] [g, h, i]]
that I would like to be mirrored accordingly:
[[g, h, i] [d, e, f] [a, b, c] [d, e, f] [g, h, i]]
And then again to yield:
[[i, h, g, h, i] [f, e, d, e, f] [c, b, a, b, c] [f, e, d, e, f] [i, h, g, h, i]]
I would like to stick to basic Python packages like numpy. Thanks in advance for any help!!