Matrix multiplication using arrays
155,736
Solution 1
You can try this code:
public class MyMatrix {
Double[][] A = { { 4.00, 3.00 }, { 2.00, 1.00 } };
Double[][] B = { { -0.500, 1.500 }, { 1.000, -2.0000 } };
public static Double[][] multiplicar(Double[][] A, Double[][] B) {
int aRows = A.length;
int aColumns = A[0].length;
int bRows = B.length;
int bColumns = B[0].length;
if (aColumns != bRows) {
throw new IllegalArgumentException("A:Rows: " + aColumns + " did not match B:Columns " + bRows + ".");
}
Double[][] C = new Double[aRows][bColumns];
for (int i = 0; i < aRows; i++) {
for (int j = 0; j < bColumns; j++) {
C[i][j] = 0.00000;
}
}
for (int i = 0; i < aRows; i++) { // aRow
for (int j = 0; j < bColumns; j++) { // bColumn
for (int k = 0; k < aColumns; k++) { // aColumn
C[i][j] += A[i][k] * B[k][j];
}
}
}
return C;
}
public static void main(String[] args) {
MyMatrix matrix = new MyMatrix();
Double[][] result = multiplicar(matrix.A, matrix.B);
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 2; j++)
System.out.print(result[i][j] + " ");
System.out.println();
}
}
}
Solution 2
Java. Matrix multiplication.
Tested with matrices of different size.
public class Matrix {
/**
* Matrix multiplication method.
* @param m1 Multiplicand
* @param m2 Multiplier
* @return Product
*/
public static double[][] multiplyByMatrix(double[][] m1, double[][] m2) {
int m1ColLength = m1[0].length; // m1 columns length
int m2RowLength = m2.length; // m2 rows length
if(m1ColLength != m2RowLength) return null; // matrix multiplication is not possible
int mRRowLength = m1.length; // m result rows length
int mRColLength = m2[0].length; // m result columns length
double[][] mResult = new double[mRRowLength][mRColLength];
for(int i = 0; i < mRRowLength; i++) { // rows from m1
for(int j = 0; j < mRColLength; j++) { // columns from m2
for(int k = 0; k < m1ColLength; k++) { // columns from m1
mResult[i][j] += m1[i][k] * m2[k][j];
}
}
}
return mResult;
}
public static String toString(double[][] m) {
String result = "";
for(int i = 0; i < m.length; i++) {
for(int j = 0; j < m[i].length; j++) {
result += String.format("%11.2f", m[i][j]);
}
result += "\n";
}
return result;
}
public static void main(String[] args) {
// #1
double[][] multiplicand = new double[][] {
{3, -1, 2},
{2, 0, 1},
{1, 2, 1}
};
double[][] multiplier = new double[][] {
{2, -1, 1},
{0, -2, 3},
{3, 0, 1}
};
System.out.println("#1\n" + toString(multiplyByMatrix(multiplicand, multiplier)));
// #2
multiplicand = new double[][] {
{1, 2, 0},
{-1, 3, 1},
{2, -2, 1}
};
multiplier = new double[][] {
{2},
{-1},
{1}
};
System.out.println("#2\n" + toString(multiplyByMatrix(multiplicand, multiplier)));
// #3
multiplicand = new double[][] {
{1, 2, -1},
{0, 1, 0}
};
multiplier = new double[][] {
{1, 1, 0, 0},
{0, 2, 1, 1},
{1, 1, 2, 2}
};
System.out.println("#3\n" + toString(multiplyByMatrix(multiplicand, multiplier)));
}
}
Output:
#1
12.00 -1.00 2.00
7.00 -2.00 3.00
5.00 -5.00 8.00
#2
0.00
-4.00
7.00
#3
0.00 4.00 0.00 0.00
0.00 2.00 1.00 1.00
Solution 3
static int b[][]={{21,21},{22,22}};
static int a[][] ={{1,1},{2,2}};
public static void mul(){
int c[][] = new int[2][2];
for(int i=0;i<b.length;i++){
for(int j=0;j<b.length;j++){
c[i][j] =0;
}
}
for(int i=0;i<a.length;i++){
for(int j=0;j<b.length;j++){
for(int k=0;k<b.length;k++){
c[i][j]= c[i][j] +(a[i][k] * b[k][j]);
}
}
}
for(int i=0;i<c.length;i++){
for(int j=0;j<c.length;j++){
System.out.print(c[i][j]);
}
System.out.println("\n");
}
}
Solution 4
Try this,
public static Double[][] multiplicar(Double A[][],Double B[][]){
Double[][] C= new Double[2][2];
int i,j,k;
for (i = 0; i < 2; i++) {
for (j = 0; j < 2; j++) {
C[i][j] = 0.00000;
}
}
for(i=0;i<2;i++){
for(j=0;j<2;j++){
for (k=0;k<2;k++){
C[i][j]+=(A[i][k]*B[k][j]);
}
}
}
return C;
}
Solution 5
try this,it may help you
import java.util.Scanner;
public class MulTwoArray {
public static void main(String[] args) {
int i, j, k;
int[][] a = new int[3][3];
int[][] b = new int[3][3];
int[][] c = new int[3][3];
Scanner sc = new Scanner(System.in);
System.out.println("Enter size of array a");
int rowa = sc.nextInt();
int cola = sc.nextInt();
System.out.println("Enter size of array b");
int rowb = sc.nextInt();
int colb = sc.nextInt();
//read and b
System.out.println("Enter elements of array a");
for (i = 0; i < rowa; ++i) {
for (j = 0; j < cola; ++j) {
a[i][j] = sc.nextInt();
}
System.out.println();
}
System.out.println("Enter elements of array b");
for (i = 0; i < rowb; ++i) {
for (j = 0; j < colb; ++j) {
b[i][j] = sc.nextInt();
}
System.out.println("\n");
}
//print a and b
System.out.println("the elements of array a");
for (i = 0; i < rowa; ++i) {
for (j = 0; j < cola; ++j) {
System.out.print(a[i][j]);
System.out.print("\t");
}
System.out.println("\n");
}
System.out.println("the elements of array b");
for (i = 0; i < rowb; ++i) {
for (j = 0; j < colb; ++j) {
System.out.print(b[i][j]);
System.out.print("\t");
}
System.out.println("\n");
}
//multiply a and b
for (i = 0; i < rowa; ++i) {
for (j = 0; j < colb; ++j) {
c[i][j] = 0;
for (k = 0; k < cola; ++k) {
c[i][j] += a[i][k] * b[k][j];
}
}
}
//print multi result
System.out.println("result of multiplication of array a and b is ");
for (i = 0; i < rowa; ++i) {
for (j = 0; j < colb; ++j) {
System.out.print(c[i][j]);
System.out.print("\t");
}
System.out.println("\n");
}
}
}
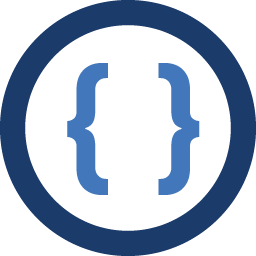
Author by
Admin
Updated on July 29, 2021Comments
-
Admin over 2 years
I'm trying to make a simple matrix multiplication method using multidimensional arrays (
[2][2]
). I'm kinda new at this, and I just can't find what it is I'm doing wrong. I'd really appreciate any help in telling me what it is. I'd rather not use libraries or anything like that, I'm mostly doing this to learn how it works. Thank you so much in advance.I'm declaring my arays in the main method as follows:
Double[][] A={{4.00,3.00},{2.00,1.00}}; Double[][] B={{-0.500,1.500},{1.000,-2.0000}};
A*B should return the identity matrix. It doesn't.
public static Double[][] multiplicar(Double[][] A, Double[][] B){ //the method runs and returns a matrix of the correct dimensions //(I actually changed the .length function to a specific value to eliminate //it as a possible issue), but not the correct values Double[][] C= new Double[2][2]; int i,j; ////I fill the matrix with zeroes, if I don't do this it gives me an error for(i=0;i<2;i++) { for(j=0;j<2;j++){ C[i][j]=0.00000; } } ///this is where I'm supposed to perform the adding of every element in //a row of A multiplied by the corresponding element in the //corresponding column of B, for all columns in B and all rows in A for(i=0;i<2;i++){ for(j=0;j<2;j++) C[i][j]+=(A[i][j]*B[j][i]); } return C; }