Maven configuration with Spring Boot & multi modules - run application in Intellij
Solution 1
You need to tell SpringBoot where to look for your controllers. Per default that only happens in sub-packages of your @SpringBootApplication
class (which will probably not include your sub module).
In order to change that you can use @ComponentScan("path.to.package")
to change the default package.
Additionally, you can use @EntityScan
to do the same for @Entity
classes that might be in your sub-module.
Solution 2
Your project structured as:
- parent
- pom.xml
- main module
- controller
- domain
- App.java (Spring Boot main class)
- pom.xml (add sub moudle to main module as dependency)
- sub module
- controllers
- domain
- pom.xm
if your App.java in a package: com.xxxx.pro,then set the sub module's package is com.xxx.pro,such as your sub module's controller is TestController.java, and the code is:
package com.xx.pro.web;
@RestController
public class TestController{
}
so, this sub moudle's TestController will be sanned by App.java.Try it on, good luck.
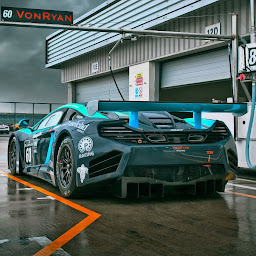
klu00
Updated on February 16, 2020Comments
-
klu00 about 4 years
I'm currently working on a REST API with Spring Boot.
I'm new to Maven and have just started coding with IDEA (don't know well this IDE yet), and I have a problem...
Here is my project structure :
- parent
- pom.xml
- main module
- controller
- domain
- App.java (Spring Boot main class)
- pom.xml
- sub module (need main module as dependency)
- controllers
- domain
- pom.xml
So when I run the project in Intellij, it starts, and I can access all URLs defined in the main module controller. But not the ones in the sub module controller... It looks like only the main module was loaded.
Here is my parent pom.xml :
<project> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.2.1.RELEASE</version> </parent> <modelVersion>4.0.0</modelVersion> <name>Test :: Test :: Parent POM</name> <groupId>test.test.test</groupId> <artifactId>project-parent</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>pom</packaging> <properties> <!-- Specify Java Compiler Version --> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> <!-- Spring --> <spring-boot.version>1.2.1.RELEASE</spring-boot.version> <!-- Sonar --> <sonar-maven-plugin.version>2.5</sonar-maven-plugin.version> <sonar.language>java</sonar.language> <sonar.core.codeCoveragePlugin>jacoco</sonar.core.codeCoveragePlugin> <sonar.dynamicAnalysis>reuseReports</sonar.dynamicAnalysis> <sonar.jacoco.reportPath>${project.basedir}/../target/jacoco.exec</sonar.jacoco.reportPath> <!-- Plugin --> <maven-surefire-plugin.version>2.18.1</maven-surefire-plugin.version> <jacoco-maven-plugin.version>0.7.3.201502191951</jacoco-maven-plugin.version> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> </properties> <modules> <module>submodule</module> <module>main</module> </modules> <build> <pluginManagement> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <version>${maven-surefire-plugin.version}</version> <configuration> <redirectTestOutputToFile>true</redirectTestOutputToFile> <includes> <include>**/*Test.java</include> <include>**/*IT.java</include> <include>**/*Story.java</include> </includes> </configuration> </plugin> </plugins> </pluginManagement> <plugins> <plugin> <groupId>org.jacoco</groupId> <artifactId>jacoco-maven-plugin</artifactId> <version>${jacoco-maven-plugin.version}</version> <configuration> <destFile>${project.basedir}/../target/jacoco.exec</destFile> </configuration> <executions> <execution> <goals> <goal>prepare-agent</goal> </goals> </execution> </executions> </plugin> <plugin> <groupId>org.codehaus.mojo</groupId> <artifactId>sonar-maven-plugin</artifactId> <version>${sonar-maven-plugin.version}</version> </plugin> </plugins> </build> </project>
Here my main module pom.xml :
<project> <parent> <artifactId>project-parent</artifactId> <groupId>test.test.test</groupId> <version>0.0.1-SNAPSHOT</version> </parent> <modelVersion>4.0.0</modelVersion> <artifactId>main</artifactId> <name>Test :: Test :: Main</name> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <commons-lang3.version>3.3.2</commons-lang3.version> <commons-codec.version>1.10</commons-codec.version> <jsr305.version>3.0.0</jsr305.version> <!-- Testing dependencies --> <http-commons.version>4.3.6</http-commons.version> <jbehave.version>3.9.5</jbehave.version> <assertj.version>1.7.1</assertj.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-rest</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency> <dependency> <groupId>com.google.code.findbugs</groupId> <artifactId>jsr305</artifactId> <version>${jsr305.version}</version> </dependency> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-lang3</artifactId> <version>${commons-lang3.version}</version> </dependency> <dependency> <groupId>commons-codec</groupId> <artifactId>commons-codec</artifactId> <version>${commons-codec.version}</version> </dependency> <!-- Test dependencies --> <dependency> <groupId>com.h2database</groupId> <artifactId>h2</artifactId> </dependency> <dependency> <groupId>postgresql</groupId> <artifactId>postgresql</artifactId> <version>9.1-901-1.jdbc4</version> </dependency> <dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>httpclient</artifactId> <version>${http-commons.version}</version> <scope>test</scope> </dependency> <dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>fluent-hc</artifactId> <version>${http-commons.version}</version> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.data</groupId> <artifactId>spring-data-commons</artifactId> </dependency> <dependency> <groupId>org.jbehave</groupId> <artifactId>jbehave-spring</artifactId> <version>${jbehave.version}</version> <scope>test</scope> </dependency> <dependency> <groupId>org.assertj</groupId> <artifactId>assertj-core</artifactId> <version>${assertj.version}</version> </dependency> </dependencies> <!-- Package as an executable jar --> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
And here the sub module pom.xml :
<project> <parent> <artifactId>project-parent</artifactId> <groupId>test.test.test</groupId> <version>0.0.1-SNAPSHOT</version> </parent> <modelVersion>4.0.0</modelVersion> <artifactId>submodule</artifactId> <name>Test :: Test :: Submodule</name> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <commons-lang3.version>3.3.2</commons-lang3.version> <commons-codec.version>1.10</commons-codec.version> <jsr305.version>3.0.0</jsr305.version> <!-- Testing dependencies --> <http-commons.version>4.3.6</http-commons.version> <jbehave.version>3.9.5</jbehave.version> <assertj.version>1.7.1</assertj.version> </properties> <dependencies> <dependency> <groupId>test.test.test</groupId> <artifactId>main</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency> </dependencies> </project>
I think that's correct, but not sure... I run the project in Intellij with Maven with config :
- working directory is root (not sub-module)
- command line
mvn spring-boot:run -Drun.arguments=--spring.profiles.active=dev -e -pl main
- property start-class with parent.main.App
Need your help to configure all that stuff to run Spring Boot with all sub-modules loaded in the IDE for dev purpose... because I readlly don't know what is wrong in my config !
Thx !
- parent
-
klu00 about 9 yearsThx for you answer. Now I just have 1 submodule but I will have much more in the future, will I need to include all paths to
@ComponentScan
? Is there a way to automatically include all controllers present in the different submodules ? -
mhlz about 9 yearsIf they all have a common root package you can just specify that and it'll scan all sub-packages as well.
-
mhlz about 9 yearsIf there's a lot of classes to scan and load this will increase the boot up time of the application of course. But that shouldn't matter too often in practice.
-
ACV over 8 yearsI don't understand why standard Spring packages are not getting picked with this approach:
Caused by: org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'webSecurityConfiguration': Injection of autowired dependencies failed;
-
mhlz over 8 years@ACV I don't really understand what you're problem is. You should ask a different question with the full stack trace.