Max character length for Read command (input)
So with bash
there are (at least) two options.
The first is read -n 5
. This may sound like it meets your needs. From the man page
-n nchars
read returns after reading nchars characters rather than
waiting for a complete line of input, but honor a delim-
iter if fewer than nchars characters are read before the
delimiter.
BUT there's a gotcha here. If the user types abcde
then the read
completes without them needing to press RETURN. This limits the results to 5 characters, but may not be a good user experience. People are used to pressing RETURN.
The second method is just to test the length of the input and complain if it's too long. We use the fact that ${#id}
is the length of the string.
This results in a pretty standard loop.
ok=0
while [ $ok = 0 ]
do
echo "Enter the id: "
read id
if [ ${#id} -gt 5 ]
then
echo Too long - 5 characters max
else
ok=1
fi
done
If you want it to be exactly 5 characters then you can change the if
test from -gt
to -eq
.
Related videos on Youtube
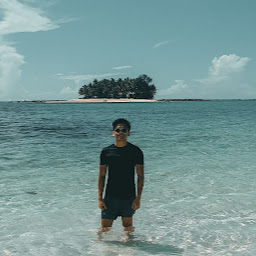
Edmhar
Updated on September 18, 2022Comments
-
Edmhar almost 2 years
I have bash script that have input commands.
echo "Enter the id: " read id
I'd like to know if there's a way I can limit the character can I input in the for
id
. I mean example he can only enter 5 characters forid
.is that possible?
Thank you.
-
Stéphane Chazelas almost 8 yearsNote that the
read -d 5
counts the characters (not bytes, and if there are bytes not forming valid characters, you may get unexpected results) before the IFS trimming and after backslash processing. For insance, if you enter<space><space>abcd
, it will storeabc
in$id
, and if you enter\a\b\c\d\e
, it will storeabcde
in$id
. To avoid IFS trimming and backslash processing, useIFS= read -rn 5 id
-
Stéphane Chazelas almost 8 yearsNote that
zsh
hasread -k n
to read n characters (long beforebash
), but it's more likeksh93
'sread -N
in that it will not stop at newline. Like for bash'sread -n
or ksh'sread -n/N
, if the input is coming from a terminal, the terminal is put out of the canonical mode, so bytes are sent as soon as they are entered, which also means things like backspace or ctrl-u won't work as usual.