Md5 Alternative in PHP?
Solution 1
SHA512 is not a terrible choice. But it won't be long before it is cracked for most lengths of passwords. Take Jeff's Advice. Use bcrypt or PBKDF2. They are resistant to EC2 or other parallel processing techniques. Probably not immune, but resistant.
The reason a better hash isn't better in this case is ABC
in MD5 becomes one value and ABC
in SHA512 becomes one value. Calculating all the 3 letter passwords is actually easier for SHA512 than it was for MD5. The key is to be hard to do. That means a slow hash, one that uses a lot of RAM, and/or one that can't be easily done on EC2.
The best choice is to NOT use passwords alone anymore at all. Decide if login is really needed for your site, and if it is, try to use a 3rd party provider first. If that's not an option consider a password + token from RSA. Only use a password alone if you have no other viable options.
When hashing a password the key is to hash(password + salt) and the salt needs to be unique per user, and should also be difficult or impossible to guess. Using the Username meets the first criteria and is better than no salt or the same salt for each user, but a unique random salt for each user is a better choice. Ideally in a separate database, if not location, with its own credentials. It should also be keyed not on the username, but on the user_id or even a hash of the user_id. You want that database to be SQL injection proof. Accepting no input from the user that isn't hashed is a good way to do that. And you would be wise to let the query itself do the hashing. Slow? Yes, and that's ALSO a good thing.
The salt itself should contain all the hard to predict data it can get. Username + User Id + Timestamp + garbage from dev/urandom + the latest tweet with the word taco in it. Longer the better. SHA512 is a good choice of hash here.
Summary: hash = bcrypt(password + salt), salt = sha512(username + user_id + timestamp + microtimestamp + dev/urandom bits + other noise).
If you are still worried after all of that. Feel free to SHA512 the bcrypted password. You'll gain the strength of SHA512, which when cracked only reveals a much harder to brute force bcrypted hash.
Solution 2
Try the php hash() function http://php.net/manual/en/book.hash.php
Example code:
$hash = hash('SHA512', $pass);
This turns this:
SHA512("The quick brown fox jumps over the lazy dog")
Into:
0x07e547d9586f6a73f73fbac0435ed76951218fb7d0c8d788a309d785436bbb642e93a252a954f23912547d1e8a3b5ed6e1bfd7097821233fa0538f3db854fee6
Further reading: http://en.wikipedia.org/wiki/SHA-2
Solution 3
MD5 is indeed not recommended anymore. Most php installations include the hash
extension. The best choice for a hash function would currently be SHA-512. You can use SHA-512 with the following function:
<?php
function sha512($string) {
return hash('sha512', $string);
}
?>
There are other alternatives with smaller output sizes if you wish, such as SHA-256
or RIPEMD-160
.
Update: Reading your updated question, I suggest the php crypt function.
Solution 4
You can use hash like this, you have plenty of algorithm to hash your data.
hash('sha256', $data);
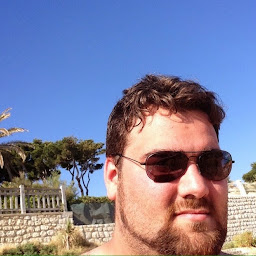
Comments
-
gregory boero.teyssier almost 2 years
What's the best alternative to the Md5 algorithm for PHP for encrypting user data like passwords and other secure data, stored in MySQL databases?
-
David Bélanger about 12 yearsFalse. sha384 is more secure : en.wikipedia.org/wiki/SHA_hash_functions
-
Chris Smith about 12 yearsMore secure than
SHA-256
, yes. More secure thanSHA-512
, no. Where did you read this? -
DampeS8N about 12 years
-
DampeS8N about 12 yearsYes, but you don't discuss which ones are more secure and why.
-
DampeS8N about 12 yearsCare to give evidence for why you disagree?