method does not override or implement a method from a supertype
Solution 1
Your Employee class has 4 abstract methods, none of which are implemented (not properly at least). Here is an example:
double caclulateWeeklyPay(); // in Employee
double calculateWeeklyPay(double w, double h) // in HourlyEmployee
The parent class should contain the same signature (which includes parameters), and should look like this:
public abstract double calculateWeeklyPay(double w, double h);
Since this appears to be homework, I will leave the rest to you.
Solution 2
Just read the error message carefully:
HourlyEmployee is not abstract and does not override abstract method resetWeek() in Employee
It clearly indicates that your Employee
class is abstract
and has an abstract method resetWeek()
.
Your class HourlyEmployee
which extends from Employee
is not abstract, so it should override all abstract methods of the super class, as a concrete class can not have any abstract methods.
The reason is that your methods in the HourlyEmployee
class have a different signature than those in the super class. So the method is not overridden, and the @Override
annotation is not accepted by the compiler
Solution 3
as your HourlyEmployee *class is not abstract* you need to implement the abstract methods declared in your EMPLOYEE abstract class. which is quite obvious. you have to implement the below methods
public abstract double caclulateWeeklyPay();
public abstract void annualRaise();
public abstract double holidayBonus();
public abstract void resetWeek();
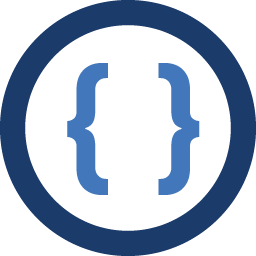
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am creating a mock employee database using inheritance and polymorphism. I am running into the following errors when trying to override the superclass methods.
HourlyEmployee is not abstract and does not override abstract method resetWeek() in Employee public class HourlyEmployee extends Employee ^ HourlyEmployee.java:43: error: method does not override or implement a method from a supertype @Override ^ HourlyEmployee.java:54: error: method does not override or implement a method from a supertype @Override ^ HourlyEmployee.java:60: error: method does not override or implement a method from a supertype @Override ^ HourlyEmployee.java:66: error: method does not override or implement a method from a supertype @Override
Here is my Employee Superclass and HourlyEmployee Subclass code
public abstract class Employee { protected String firstName; protected String lastName; protected char middleInitial; protected boolean fulltime; private char gender; private int employeeNum; public Employee (String fn, String ln, char m, char g, int empNum, boolean ft) { firstName = fn; lastName = ln; middleInitial = m; gender = g; employeeNum = empNum; fulltime = ft; } public int getEmployeeNumber() { return employeeNum; } public void setEmployeeNumber(int empNum) { while (empNum <= 10000 && empNum >= 99999) { System.out.print ("Invalid input, please try again: "); } if (empNum >= 10000 && empNum <= 99999) { employeeNum = empNum; } } public String getFirstName() { return firstName; } public String getLastName() { return lastName; } public char checkGender(char g) { if (g != 'M' || g != 'F') { g = 'F'; } return g; } public char getGender() { return gender; } @Override public boolean equals(Object e2) { if (this.employeeNum == ((Employee)e2).employeeNum) { return true; } else { return false; } } @Override public String toString() { return employeeNum + "\n" + lastName + ", " + firstName + "\n" + "Gender:" + gender + "\n" + "Status:" + fulltime + "\n"; } public abstract double caclulateWeeklyPay(); public abstract void annualRaise(); public abstract double holidayBonus(); public abstract void resetWeek(); }
Here is the HourlyEmployee subclass
public class HourlyEmployee extends Employee { private double wage; private double hoursWorked; private double additionalHours; public HourlyEmployee(String fn, String ln, char m, char g, int empNum, boolean ft, double w, double h, double ahours) { super (fn, ln, m, g, empNum, ft); wage = w; hoursWorked = h; hoursWorked = 0.0; additionalHours = ahours; } @Override public String toString() { return this.getEmployeeNumber() + "\n" + lastName + ", " + firstName + middleInitial + "\n" + "Gender: " + this.getGender() + "\n" + "Status: " + fulltime + "\n" + "Wage: " + wage + "\n" + "Hours Worked: " + hoursWorked + "\n"; } //@Override public double calculateWeeklyPay(double w, double h) { if (h > 40) { w = w * 2; } return w * h; } //@Override public double annualRaise(double w) { return (w * .05) + w; } //@Override public double holidayBonus(double w) { return w * 40; } //@Override public double resetWeek(double h) { h = 0.0; return h; } public double plusHoursWorked(double h, double ahours) { while (h <= 0) { System.out.println("Invalid value entered, please try again"); } h += ahours; return h; } }